用Deflate流压缩/解压缩文件:
Deflate流压缩:
using System.IO;
using System.IO.Compression;
string sourceFile=@"C:\1.txt";
string destinationFile=@"C:\1.bin";
private const long BUFFER_SIZE = 20480;
if ( File.Exists ( sourceFile ))
{
FileStream sourceStream = null;
FileStream destinationStream = null;
DeflateStream compressedStream = null;
try
{
// Read the bytes from the source file into a byte array
sourceStream = new FileStream ( sourceFile, FileMode.Open, FileAccess.Read, FileShare.Read );
// Open the FileStream to write to
destinationStream = new FileStream ( destinationFile, FileMode.OpenOrCreate, FileAccess.Write );
// Create a compression stream pointing to the destiantion stream
compressedStream = new DeflateStream ( destinationStream, CompressionMode.Compress, true );
long bufferSize = sourceStream.Length < BUFFER_SIZE ? sourceStream.Length : BUFFER_SIZE;
byte[] buffer = new byte[bufferSize];
int bytesRead = 0;
long bytesWritten = 0;
while ((bytesRead = sourceStream.Read(buffer, 0, buffer.Length)) != 0)
{
compressedStream.Write(buffer, 0, bytesRead);
bytesWritten += bufferSize;
}
}
catch ( ApplicationException ex )
{
Console.WriteLine(ex.Message);
}
finally
{
// Make sure we allways close all streams
if ( sourceStream != null )
sourceStream.Close ( );
if ( compressedStream != null )
compressedStream.Close ( );
if ( destinationStream != null )
destinationStream.Close ( );
}
}
Deflate流解压缩
using System.IO;
using System.IO.Compression;
string sourceFile=@"C:\1.bin";
string destinationFile=@"C:\1.txt";
private const long BUFFER_SIZE = 20480;
// make sure the source file is there
if (File.Exists ( sourceFile ))
{
FileStream sourceStream = null;
FileStream destinationStream = null;
DeflateStream decompressedStream = null;
byte[] quartetBuffer = null;
try
{
// Read in the compressed source stream
sourceStream = new FileStream ( sourceFile, FileMode.Open );
// Create a compression stream pointing to the destiantion stream
decompressedStream = new DeflateStream ( sourceStream, CompressionMode.Decompress, true );
// Read the footer to determine the length of the destiantion file
quartetBuffer = new byte[4];
int position = (int)sourceStream.Length - 4;
sourceStream.Position = position;
sourceStream.Read ( quartetBuffer, 0, 4 );
sourceStream.Position = 0;
int checkLength = BitConverter.ToInt32 ( quartetBuffer, 0 );
byte[] buffer = new byte[checkLength + 100];
int offset = 0;
int total = 0;
// Read the compressed data into the buffer
while ( true )
{
int bytesRead = decompressedStream.Read ( buffer, offset, 100 );
if ( bytesRead == 0 )
break;
offset += bytesRead;
total += bytesRead;
}
// Now write everything to the destination file
destinationStream = new FileStream ( destinationFile, FileMode.Create );
destinationStream.Write ( buffer, 0, total );
// and flush everyhting to clean out the buffer
destinationStream.Flush ( );
}
catch ( ApplicationException ex )
{
Console.WriteLine(ex.Message, "解压文件时发生错误:");
}
finally
{
// Make sure we allways close all streams
if ( sourceStream != null )
sourceStream.Close ( );
if ( decompressedStream != null )
decompressedStream.Close ( );
if ( destinationStream != null )
destinationStream.Close ( );
}
}
本文摘自深度开源:https://www.open-open.com/code/view/1456621729812,侵删。
相关阅读 >>
更多相关阅读请进入《Deflate》频道 >>
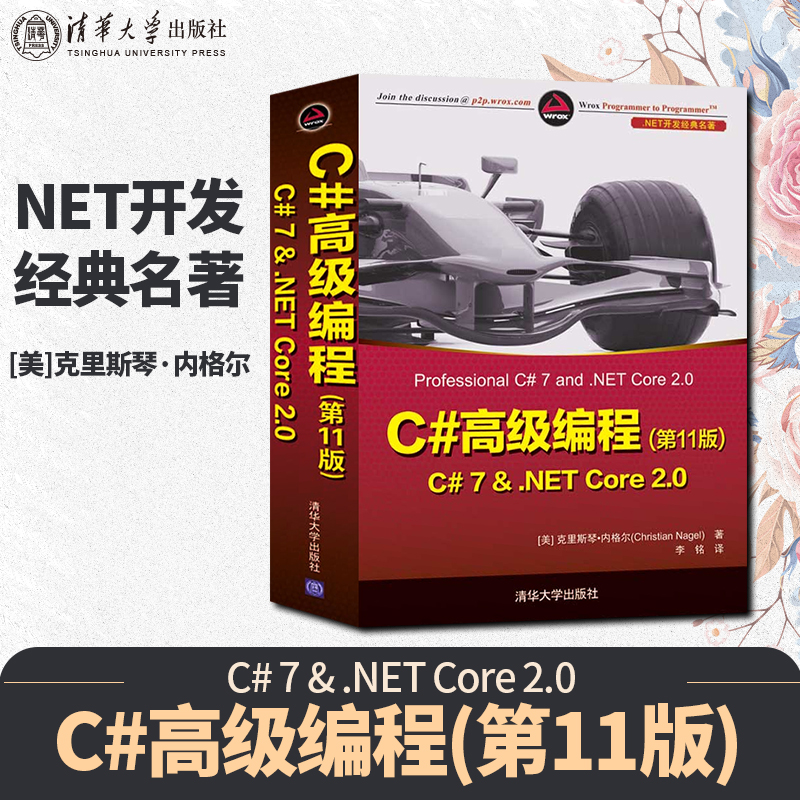
C#高级编程(第11版) C# 7 & .NET Core 2.0(.NET开发经典名著)
作者:[美]克里斯琴·内格尔(Christian Nagel)著。出版时间:2019年3月。