我们的demo Rust 应用程序 从一个文件中读取。注意这里不需要 #{wasm_bindgen]
,因为 WebAssembly 程序的输入和输出数据现在由 STDIN
和 STDOUT
传递。
use std::env;
use std::fs::File;
use std::io::{self, BufRead};
fn main() {
// Get the argv.
let args: Vec<String> = env::args().collect();
if args.len() <= 1 {
println!("Rust: ERROR - No input file name.");
return;
}
// Open the file.
println!("Rust: Opening input file \"{}\"...", args[1]);
let file = match File::open(&args[1]) {
Err(why) => {
println!("Rust: ERROR - Open file \"{}\" failed: {}", args[1], why);
return;
},
Ok(file) => file,
};
// Read lines.
let reader = io::BufReader::new(file);
let mut texts:Vec<String> = Vec::new();
for line in reader.lines() {
if let Ok(text) = line {
texts.push(text);
}
}
println!("Rust: Read input file \"{}\" succeeded.", args[1]);
// Get stdin to print lines.
println!("Rust: Please input the line number to print the line of file.");
let stdin = io::stdin();
for line in stdin.lock().lines() {
let input = line.unwrap();
match input.parse::<usize>() {
Ok(n) => if n > 0 && n <= texts.len() {
println!("{}", texts[n - 1]);
} else {
println!("Rust: ERROR - Line \"{}\" is out of range.", n);
},
Err(e) => println!("Rust: ERROR - Input \"{}\" is not an integer: {}", input, e),
}
}
println!("Rust: Process end.");
}
复制代码
使用 rustwasmc 工具将应用程序编译为 WebAssembly。
$ cd rust_readfile
$ rustwasmc build
# The output file will be pkg/rust_readfile.wasm
复制代码
Golang 源代码运行在 WasmEdge 中 WebAssembly 函数,如下:
package main
import (
"os"
"github.com/second-state/WasmEdge-go/wasmedge"
)
func main() {
wasmedge.SetLogErrorLevel()
var conf = wasmedge.NewConfigure(wasmedge.REFERENCE_TYPES)
conf.AddConfig(wasmedge.WASI)
var vm = wasmedge.NewVMWithConfig(conf)
var wasi = vm.GetImportObject(wasmedge.WASI)
wasi.InitWasi(
os.Args[1:], /// The args
os.Environ(), /// The envs
[]string{".:."}, /// The mapping directories
[]string{}, /// The preopens will be empty
)
/// Instantiate wasm. _start refers to the main() function
vm.RunWasmFile(os.Args[1], "_start")
vm.Delete()
conf.Delete()
}
复制代码
接下来,让我们使用 WasmEdge Golang SDK 构建 Golang 应用程序。
$ go get -u github.com/second-state/WasmEdge-go
$ go build
复制代码
运行 Golang 应用。
$ ./read_file rust_readfile/pkg/rust_readfile.wasm file.txt
Rust: Opening input file "file.txt"...
Rust: Read input file "file.txt" succeeded.
Rust: Please input the line number to print the line of file.
# Input "5" and press Enter.
5
# The output will be the 5th line of `file.txt`:
abcDEF___!@#$%^
# To terminate the program, send the EOF (Ctrl + D).
^D
# The output will print the terminate message:
Rust: Process end.
复制代码
接下来
本文中,我们展示了在 Golang 应用程序中嵌入 WebAssembly 函数的两种方法:嵌入一个 WebAssembly 函数以及嵌入一个完整的程序。 更多示例可以参考 WasmEdge-go-examples GitHub repo。
下一篇文章,我们将研究将 AI 推理(图像识别)函数嵌入到基于 Golang 的实时流数据处理框架的完整示例。这在智能工厂和汽车中有实际应用。
本文来自:掘金
感谢作者:Michael_Yuan
查看原文:通过 WasmEdge 嵌入WebAssembly 函数扩展 Golang 应用
相关阅读 >>
更多相关阅读请进入《Go》频道 >>
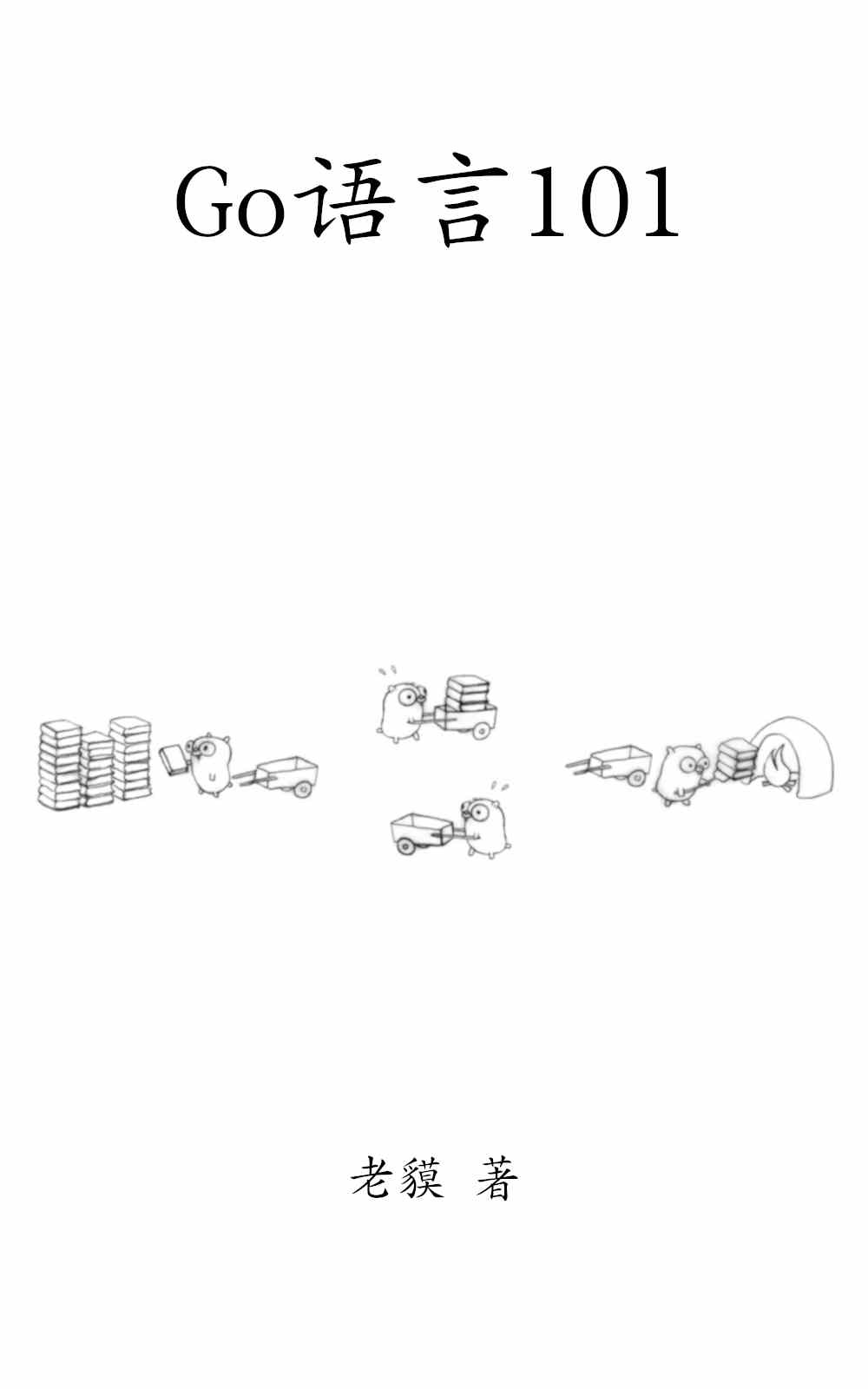
Go语言101
一个与时俱进的Go编程知识库。