1. 判断数据文件是否存在
/// <summary> /// 检查数据库是否存在不存在创建 /// </summary> /// <returns></returns> public static bool CheckDataBase() { try { //判断数据文件是否存在 bool dbExist = File.Exists("mesclient.sqlite"); if (!dbExist) { SQLiteConnection.CreateFile("mesclient.sqlite"); } return true; } catch (Exception) { return false; } }
2. 判断表是否存在
/// <summary> /// 检查数据表是否存在,不存在创建 /// </summary> /// <returns></returns> public static bool CheckDataTable(string connStr) { try { using (SQLiteConnection conn = new SQLiteConnection(connStr)) using (SQLiteCommand cmd = conn.CreateCommand()) { conn.Open(); cmd.CommandText = "SELECT count(*) FROM sqlite_master WHERE type = 'table' AND name = 'serverinfo'"; object ob = cmd.ExecuteScalar(); long tableCount = Convert.ToInt64(ob); if (tableCount == 0) { //创建表 cmd.CommandText = @" BEGIN; create table serverinfo (Id INTEGER PRIMARY KEY AUTOINCREMENT,Name TEXT, Url text,DelayTime integer,UsageCounter INTEGER, Status integer,CreateTime DATETIME); CREATE UNIQUE INDEX idx_serverInfo ON serverinfo (Name); COMMIT; "; //此语句返回结果为0 int rowCount = cmd.ExecuteNonQuery(); return true; } else if (tableCount > 1) { return false; } else { return true; } } } catch (Exception ex) { return false; } }
3. 查询
string sql = "SELECT * FROM serverinfo WHERE Name =@ServerName AND Url = @Url and date(CreateTime)=date(@Date);"; Dictionary<string, object> parameters = new Dictionary<string, object>(); parameters.Add("ServerName",endpointElement.Name); parameters.Add("Url", endpointElement.Address); parameters.Add("Date", DateTime.Now.ToString("yyyy-MM-dd")); DataTable dt=SqliteHelper.ExecuteQuery(connStr, sql, parameters); if (dt.Rows.Count>0) { UsageCounter = dt.Rows[0].Field<long>("UsageCounter"); GetTime = dt.Rows[0].Field<DateTime>("CreateTime"); }
4. 新增/修改
//存在更新,不存在插入 string updateSql = "REPLACE INTO serverinfo(Name,Url,DelayTime,UsageCounter, Status,CreateTime) VALUES(@Name,@Url,@DelayTime,@UsageCounter,@Status, @CreateTime)"; Dictionary<string, object> ups = new Dictionary<string, object>(); ups.Add("Name", name); ups.Add("Url", url); ups.Add("DelayTime", delayTime); ups.Add("UsageCounter", usageCounter); ups.Add("Status", status); ups.Add("CreateTime", DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss")); int count= SqliteHelper.ExecuteNonQuery(connStr, updateSql, ups); if (count>0) { return true; } else { return false; }
5. 删除
//删除记录 string updateSql = "DELETE FROM serverinfo where content=@Content and flag=@Flag;"; Dictionary<string, object> updateParameters = new Dictionary<string, object>(); updateParameters.Add("Content", Content); updateParameters.Add("Flag", Flag); int count = SqliteHelper.ExecuteNonQuery(connStr, updateSql, updateParameters); if (count > 0) { return true; } else { return false; }
四、参考文章
- Create SQLite Database and table
- Writing to a SQLite Database in C#
- SQLite with VS2012 and .NET 4.5 — ANY CPU Build
- how to check if a table exists in C#
- SQLite auto increment issue
- Inserting a date to SQLite
- SQLite REPLACE Statement
- sqlite select with condition on date
- Using SQLite how do I index columns in a CREATE TABLE statement?
总结
以上就是这篇文章的全部内容了,希望本文的内容对大家的学习或者工作具有一定的参考学习价值,如果有疑问大家可以留言交流,谢谢大家对的支持。
标签:SQLite
相关阅读 >>
android通过jxl读excel存入Sqlite3数据库
android平台的sql注入漏洞浅析(一条短信控制你的手机)
更多相关阅读请进入《Sqlite》频道 >>
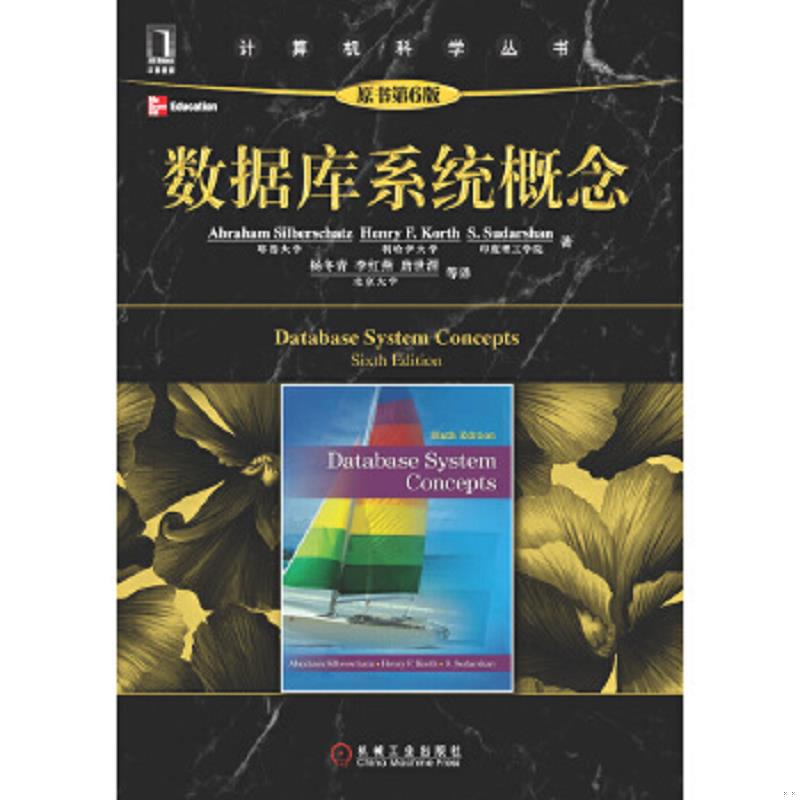
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。