本文摘自php中文网,作者不言,侵删。
本篇文章给大家带来的内容是关于python中正则表达式的详细介绍,有一定的参考价值,有需要的朋友可以参考一下,希望对你有所帮助。
正则
re = regular experssion
re 模块使 Python 语言拥有全部的正则表达式功能。
compile 函数根据一个模式字符串和可选的标志参数生成一个正则表达式对象。该对象拥有一系列方法用于正则表达式匹配和替换。
作用: 对于字符串进行处理, 会检查这个字符串内容是否与你写的正则表达式匹配
如果匹配, 拿出匹配的内容;
如果不匹配, 忽略不匹配内容;
编写正则的规则
pattern 匹配的正则表达式
string 要匹配的字符串
三种查找方法
1 2 3 4 5 6 7 8 | import re
str = 'hello sheen,hello cute.'
pattern_1 = r 'hello'
pattern_2 = r 'sheen'
print (re.findall(pattern_1,str)) #[ 'hello' , 'hello' ]
print (re.findall(pattern_2,str)) #[ 'sheen' ]
|
match尝试从字符串的起始位置开始匹配,
如果起始位置没有匹配成功, 返回一个None;
如果起始位置匹配成功, 返回一个对象;
1 2 3 4 5 6 7 8 9 | import re
str = 'hello sheen,hello cute.'
pattern_1 = r 'hello'
pattern_2 = r 'sheen'
print (re.match(pattern_1,str)) #<_sre.SRE_Match object; span=(0, 5), match= 'hello' >
print (re.match(pattern_1,str).group()) #返回match匹配的字符串内容,hello
print (re.match(pattern_2,str)) #None
|
search会扫描整个字符串, 只返回第一个匹配成功的内容;
1 2 3 4 5 6 7 8 9 10 | import re
str = 'hello sheen,hello cute.'
pattern_1 = r 'hello'
pattern_2 = r 'sheen'
print (re.search(pattern_1,str)) #<_sre.SRE_Match object; span=(0, 5), match= 'hello' >
print (re.search(pattern_1,str).group()) #hello
print (re.search(pattern_2,str)) #<_sre.SRE_Match object; span=(6, 11), match= 'sheen' >
print (re.search(pattern_2,str).group()) #sheen
|
特殊字符类
.: 匹配除了\n之外的任意字符; [.\n]
\d: digit--(数字), 匹配一个数字字符, 等价于[0-9]
\D: 匹配一个非数字字符, 等价于[^0-9]
\s: space(广义的空格: 空格, \t, \n, \r), 匹配单个任何的空白字符;
\S: 匹配除了单个任何的空白字符;
\w: 字母数字或者下划线, [a-zA-Z0-9_]
\W: 除了字母数字或者下划线, [^a-zA-Z0-9_]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | import re
# .
print (re.findall(r '.' , 'sheen\nstar\n' )) #[ 's' , 'h' , 'e' , 'e' , 'n' , 's' , 't' , 'a' , 'r' ]
#\d#\D
print (re.findall(r '\d' , '当前声望30' )) #[ '3' , '0' ]
print (re.findall(r '\D' , '当前声望30' )) #[ '当' , '前' , '声' , '望' ]
#\s#\S
print (re.findall(r '\s' , '\n当前\r声望\t为30' )) #[ '\n' , '\r' , '\t' ]
print (re.findall(r '\S' , '\n当前\r声望\t为30' )) #[ '当' , '前' , '声' , '望' , '为' , '3' , '0' ]
#\w#\W
print (re.findall(r '\w' , 'lucky超可爱!!' )) #[ 'l' , 'u' , 'c' , 'k' , 'y' , '超' , '可' , '爱' ]
print (re.findall(r '\W' , 'lucky超可爱!!' )) #[ '!' , '!' ]
|
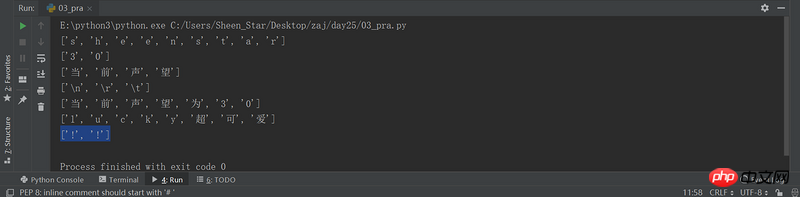
指定字符出现次数
匹配字符出现次数:
*: 代表前一个字符出现0次或者无限次; d*, .*
+: 代表前一个字符出现一次或者无限次; d+
?: 代表前一个字符出现1次或者0次; 假设某些字符可省略, 也可以不省略的时候使用
第二种方式:
{m}: 前一个字符出现m次;
{m,}: 前一个字符至少出现m次; * == {0,}; + ==={1,}
{m,n}: 前一个字符出现m次到n次; ? === {0,1}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | import re
#* 代表前一个字符出现0次或者无限次
print (re.findall(r 's*' , 'sheenstar' )) #[ 's' , '' , '' , '' , '' , 's' , '' , '' , '' , '' ]
print (re.findall(r 's*' , 'hello' )) #[ '' , '' , '' , '' , '' , '' ]
#+ 代表前一个字符出现一次或者无限次
print (re.findall(r 's+' , 'sheenstar' )) #[ 's' , 's' ]
print (re.findall(r 's+' , 'hello' )) #[]
# ? 代表前一个字符出现1次或者0次
print (re.findall(r '188-?' , '188 6543' )) #[ '188' ]
print (re.findall(r '188-?' , '188-6543' )) #[ '188-' ]
print (re.findall(r '188-?' , '148-6543' )) #[]
# 匹配电话号码
pattern = r '\d{3}[\s-]?\d{4}[\s-]?\d{4}'
print (re.findall(pattern, '188 0123 4567' )) #[ '188 0123 4567' ]
print (re.findall(pattern, '188-0123-4567' )) #[ '188-0123-4567' ]
print (re.findall(pattern, '18801234567' )) #[ '188-0123-4567' ]
|
练习--匹配IP
可以从网上搜索正则表达式生成器,使用别人写好的规则,自己测试。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | import re
# | 表示或者
pattern = r '(25[0-5]|2[0-4]\d|[0-1]\d{2}|[1-9]?\d)\.(25[0-5]|2[0-4]\d|[0-1]\d{2}|[1-9]?\d)\.(25[0-5]|2[0-4]\d|[0-1]\d{2}|[1-9]?\d)\.(25[0-5]|2[0-4]\d|[0-1]\d{2}|[1-9]?\d)$'
print (re.findall(pattern, '172.25.254.34' )) #[( '172' , '25' , '254' , '34' )]
matchObj_1 = re.match(pattern, '172.25.254.34' )
if matchObj_1:
print ( '匹配项:' ,matchObj_1.group()) #172.25.254.34
else :
print ( '未找到匹配项' )
matchObj_2 = re.match(pattern, '172.25.254.343' )
if matchObj_2:
print ( '匹配项:' ,matchObj_2.group())
else :
print ( '未找到匹配项' )
|
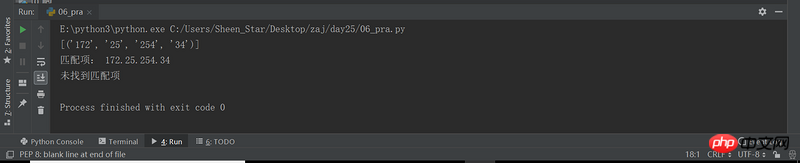
以上就是python中正则表达式的详细介绍的详细内容,更多文章请关注木庄网络博客!!
相关阅读 >>
Python中迭代器与迭代器切片的详细介绍
Python中的类是什么?如何创建类?
Python可以开发什么
Python对字符串实现重操作方法讲解
Python基础流程控制的介绍(代码示例)
Pythonn如何访问本地html
Python如何重命名文件
Python中的条件判断语句基础学习
理解Python的全局变量和局部变量
Python如何计算平方和
更多相关阅读请进入《Python》频道 >>
人民邮电出版社
python入门书籍,非常畅销,超高好评,python官方公认好书。
转载请注明出处:木庄网络博客 » python中正则表达式的详细介绍