本文摘自网络,作者tangxia,侵删。
Syracuse University CIS 657: Principles of Operating Systems Spring 2021 PA-5: Nachos System Calls for File I/O and Multi-programming Total points: 100 pts Due on Apr May 8, 2021 at 11:59 PM Instructor: Endadul Hoque Important Reminders Academic Integrity: Review the academic integrity policy (from the syllabus) and the course honor pledge; you submitted a signed pledge at the beginning of the semester. For this programming assignment and the future ones, we will use moss (https://theory.stanford.edu/~aiken/moss/), a system for detecting software similarity, extensively to detect plagiarism among this semester’s submissions as well as previous years’ submissions (if applicable). Remember that violating academic integrity policy will significantly jeopardize your grade as you agreed on the honor pledge. The first step for success: As with every lab/PA handout/description, I strongly urge you to read every word very carefully. You may be able to find answers to many questions about each task by carefully reading this document. FThe late submission policy is not applicable for this PA. Logistics
- This lab must be done individually, not in groups.
- This PA expects that you are already familiar with the Nachos code base and know how to build and run nachos and execute test programs. All these aspects were covered in the lab on Nachos.
- For this programming assignment (PA-5), you have to download an archive called student-pa5.tar.gz from Blackboard. Upon extraction, you will see a directory named student/, which contains nachos/code/ directory. You have to add to and/or edit the existing Nachos source code.
- For all the following tasks (Task-1, ...), you have to use the Linux server (lcs-vc-cis486.syr.edu) dedicated for this course. nachos will not compile and run on a different machine.
- Unless stated otherwise, you MUST NOT modify/edit/rename/move other existing files under the student/ directory.
- Unless stated otherwise, you MUST NOT use any additional C/C++ library (e.g., math library) that requires modification to the compilation commands included in the existing Makefiles.
- For the following task, you have to use the Linux server (lcs-vc-cis486.syr.edu) dedicated for this course. You must know how to remotely login to that machine, how to use terminal and how to copy files back and forth between your computer and the Linux server. Page 1 of 11
- For this PA, you have to write a report as well. Your answers must be typed. Only one PDF file is allowed for submission.
- Each task explains what you have to do and “✪ [R-1]” dictates what you have to report about the task.
- You must follow the submission instructions. How to obtain the skeleton/source code for this PA? Obtain student-pa5.tar.gz from Blackboard. Unpack the package to find the skeleton code required for this PA. The extracted directory is named student/. $ tar xzf student-pa5.tar.gz Grading Please make sure you follow the instructions provided throughout this handout; otherwise your assignment will not be graded properly. Not following instructions will result in loss of points. • You must not modify/edit/rename/move other existing files/directories in student/ or in its sub-directories. • Your solutions must execute on the Linux machine (lcs-vc-cis486.syr.edu), otherwise your solutions will not be considered for grading. • You must not change the order of the tasks on your report. • Your must follow the submission instructions, or it will not be graded properly. Submission Instructions You have to submit (a) code and (b) report using Blackboard. Please follow the instructions shown below to prepare your submission. Source code Follow these instructions when you are ready to submit.
- First clean up your nachos/code/ directory as follows:
$ cd student/nachos/code/
$ cd build.linux/
$ make distclean
Now clean up test-pa directory
$ cd ../test-pa/ $ make distclean - Go to (or change directory cd into) your student/ directory. Now student/ should be your current
working directory. To verify your current directory, try this
$ basename
pwd
student Page 2 of 11 - Use your netid to create a compressed archive (
-pa5.tar.gz) of your solution directory. For instance, if your netid is johndoe, create a compressed archive as follows: $ cd .. # moving to the parent directory $ mv student/ johndoe-pa5/ $ tar czf johndoe-pa5.tar.gz johndoe-pa5/ - To verify the content of your compressed archive, try “tar tvzf
-pa5.tar.gz”. - oFinally, download your compressed archive file (
-pa5.tar.gz). You have to submit this package. Report • You have to write a report. Your answer must be typed. You can use any typesetting program (e.g., Word, LATEX), but you have to convert the document to a PDF. Only one PDF file is allowed for submission. • Mention your full name and SU NetID on the PDF. • Use Blackboard to submit your PDF. Use the Blackboard submission link for submitting two files: (a) your code package ( -pa5.tar.gz) and (b) report (in PDF). List of Tasks for this PA 1 Implement System Calls for File I/O (40 pts) 4 2 Nachos Multi-programming (60 pts) 5 2.1 Non-preemptive and Preemptive Scheduling (10 pts) . . . . . . . . . . . . . . . . . . . . . . . . . . . 5 2.2 Preemptive Multi-programming (20 pts) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7 2.3 System Calls for Multi-programming (30 pts) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9 A Some Useful Links 11 o All the tasks must be done on the lcs-vc-cis486.syr.edu, because nachos will not compile and run on a different machine.For example, if your implementation fails test cases, we will look into your implementation and grade both implementation and testing holistically. Page 3 of 11 Task 1 Implement System Calls for File I/O (40 pts) You may want to revisit PA-4 and your solution to refresh memory on how to implement a system call. For this task, you have to implement the following system calls that the Nachos user programs can utilize for file I/O operations. [Hint: It is recommended that you implement these syscalls in the same order presented below.] - int Create(char *name): This system call creates a Unix file with the name given by the parameter “name”, and returns 1 if successful, and -1 if not, to the user program. Implement another function that extracts the file name from the user program memory so that you can re-use the name extraction function for other Nachos system calls, call to implement this Nachos system call in exception.cc. (See the documentation of Unix/Linux open() syscall) [Hint: Implement this system call first. The Nachos Open() system call implementation is very similar. ]
- OpenFileId Open(char *name): This system call opens the Unix file “name” and returns an “OpenFileId” (aka, a file descriptor) if the file (“name”) exists. This OpenFileId can be used to read from and write to the file. If the file doesn’t exist, Open() returns -1 to the user program to indicate an error. call to implement this Nachos system call in exception.cc. (See the documentation of Unix/Linux open() syscall) Hint: You don’t have to maintain a separate mapping between Nachos OpenFileIds and Unix file descriptors. Instead you can simple return the same file descriptor that the Unix open(...) syscall returns.
- int Write(char *buffer, int size, OpenFileId id): Utilize your implementation of Write() from PA-4 and extend it so that it can now write to an already open Unix file (specified by id) as well as print out to the screen. This function returns the number of bytes actually written or -1 on failure. call to implement this Nachos system call in exception.cc. (See the documentation of Unix/Linux write() syscall)
- int Read(char *buffer, int size, OpenFileId id): This system call reads size bytes from the open file (specified by id) and stores them into buffer. On success, it returns the number of bytes actually read from the file (note: it can be 0 too), and on failure, it returns -1. For this task, implement only the case for reading from a file; you don’t have to implement the case for reading from ConsoleInput (i.e., keyboard input). call to implement this Nachos system call in exception.cc. (See the documentation of Unix/Linux read() syscall)
- int Close(OpenFileId id): Close the file specified by id. It returns 1 on success and -1 on failure. call to implement this Nachos system call in exception.cc. (See the documentation of Unix/Linux close() syscall) What to report for Task 1? Try the list of tasks below in the order and provide answer/screenshot as stated. Do not forget to re-build nachos if required and run it from build.linux/ [R-1] (4 × 5 = 20 pts) For each system call mentioned above, provide at least one screenshot of your implementation. Your screenshots should be legible. Therefore, if the implementation of a system call requires more than one screenshots, please provide them and mark them appropriately. [Hint: There should be at least 5 screenshots.] Page 4 of 11 [R-2] ((1 + 1 + 1) × 4 = 12 pts) Build the test programs in test-pa/ if needed. Build nachos in build.linux/. test-pa/ includes four test files for the file systems calls: file-test0.c, file-test1.c, file-test2.c and file-test3.c. Explain what should be the results of running each of these test programs, file-test0.c, file-test1.c, file-test2.c and file-test3.c. Explain what features of the file system calls are tested with each test program. Run these test programs with Nachos. Provide a screenshot of the output of running each program, and provide your explanations. Some of these programs are expected to create some local files. Make sure to include a screenshot of each file along with the program output. [R-3] (8 pts) Write a new test program file-test4.c of your own to test the returned values of calling Read() and Close(). For each of these system calls, your program should demonstrate one success case and one failure case. Your program should print out the appropriate messages on the screen as shown below: • For Read(): – Print “Read(): Successful: read x bytes”, where x is the value returned by Read() on success. – Print “Read(): Failed: returned y”, where y is the value returned by Read() on failure. • For Close(): – Print “Close(): Successful: returned x”, where x is the value returned by Close() on success. – Print “Close(): Failed: returned y”, where y is the value returned by Close() on failure. Your file-test4.c should printout the returned values (i.e., integers) as shown above. Refer to file-test3.c. Your file-test4.c needs to have a similar function to convert an integer (i.e., positive, 0, negative) to a string. 请加QQ:99515681 或邮箱:99515681@qq.com WX:codehelp
相关阅读 >>
更多相关阅读请进入《Go》频道 >>
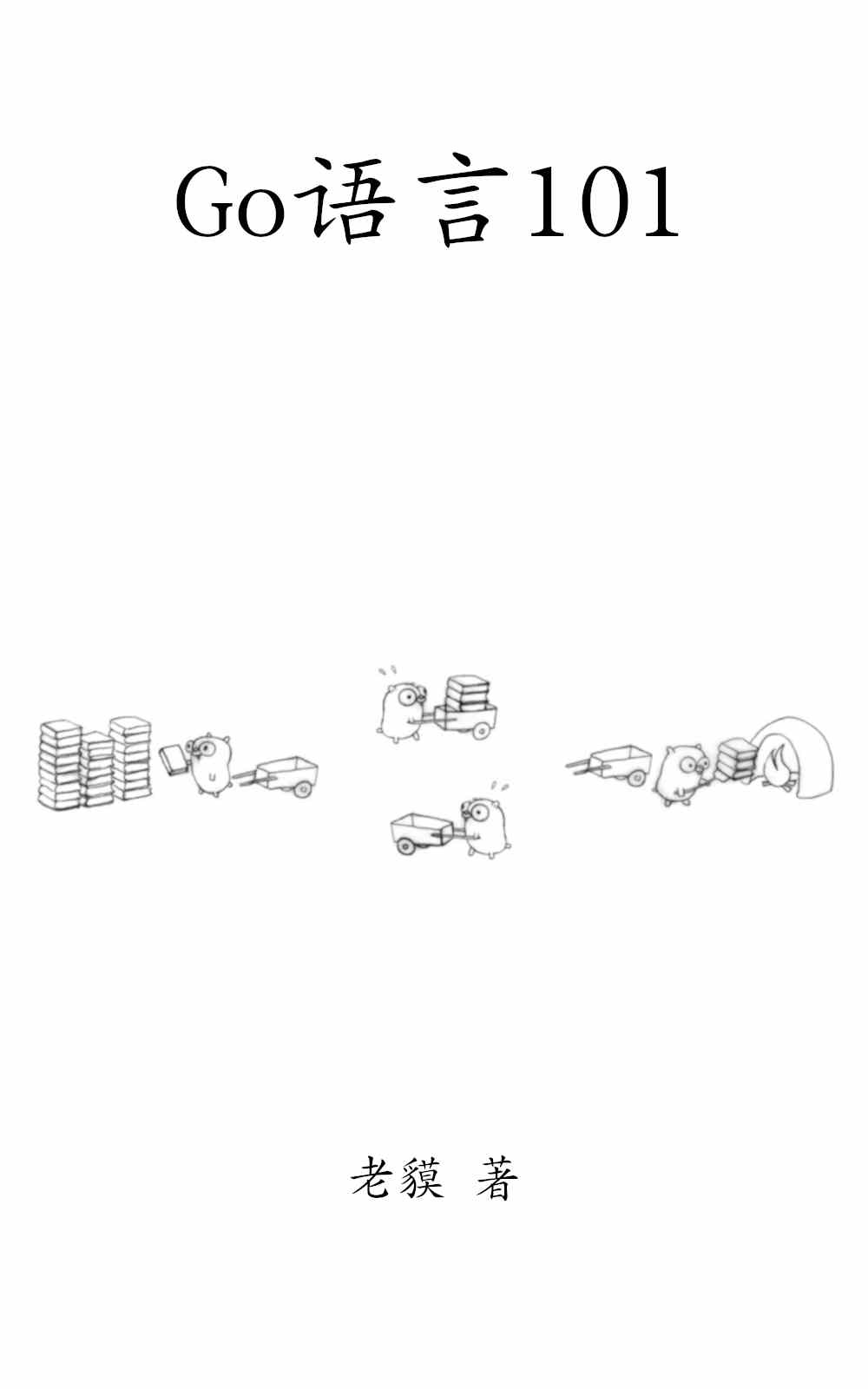
Go语言101
一个与时俱进的Go编程知识库。