本文摘自网络,作者,侵删。
结构体和Json相互转换
当Golang要为App或者小程序提供Api接口数据时,涉及到结构体和Json之间的相互转换
Golang序列化是指把结构体数据转换成Json格式的字符串;Golang Json的反序列化是指把Json数据转化成Golang中的结构体对象
Golang中的序列化和反序列化主要通过
"encoding/json"
包中的json.Marshal()和json.Unmarsual()
- 序列化
结构体中私有属性不能被json包访问(首字母必须大写)
package main
import (
"fmt"
"encoding/json"
)
type Student struct {
Id int
Gender string
Name string //私有属性不能被json包访问(首字母必须大写)
Sno string
}
func main() {
var s1 = Student {
Id : 12,
Gender : "男",
Name : "李四",
Sno : "s001",
}
fmt.Printf("%#v\n", s1)
//main.Student{Id:12, Gender:"男", Name:"李四", Sno:"s001"}
jsonByte, _ := json.Marshal(s1)
jsonStr := string(jsonByte)
fmt.Printf("%v", jsonStr)
//{"Id":12,"Gender":"男","Name":"李四","Sno":"s001"}
}
- 反序列化
type Student struct {
ID int
Gender string
Name string
Sno string
}
func main() {
var str = `{"Id":12,"Gender":"男","Name":"李四","Sno":"s001"}`
var s1 Student
err := json.Unmarshal([]byte(str),&s1)
if err != nil {
fmt.Println(err)
}
fmt.Printf("%#v\n", s1)
fmt.Println(s1.Name)
//main.Student{ID:12, Gender:"男", Name:"李四", Sno:"s001"}
//李四
}
- 嵌套结构体的序列化
type Student struct {
Id int
Gender string
Name string
}
type Class struct {
Title string
Students []Student
}
func main(){
c := Class {
Title : "01班",
Students : make([]Student, 0),
}
for i := 1; i <= 10; i++ {
s := Student {
Id:i,
Gender:"男",
Name:fmt.Sprintf("stu_%v", i),
}
c.Students = append(c.Students, s)
}
fmt.Println(c)
//{01班 [{1 男 stu_1} {2 男 stu_2} {3 男 stu_3} {4 男 stu_4}
//{5 男 stu_5} {6 男 stu_6} {7 男 stu_7} {8 男 stu_8}
//{9 男 stu_9} {10 男 stu_10}]}
strByte, err := json.Marshal(c)
if err != nil {
fmt.Println(err)
} else {
fmt.Println(string(strByte))
}
//{"Title":"01班","Students":[{"Id":1,"Gender":"男","Name":"stu_1"},
//{"Id":2,"Gender":"男","Name":"stu_2"},……,{"Id":10,"Gender":"男","Name":"stu_10"}]}
}
- 嵌套结构体的反序列化
type Student struct {
Id int
Gender string
Name string
}
type Class struct {
Title string
Students []Student
}
func main() {
str := `{"Title":"01班","Students":[{"Id":1,"Gender":"男","Name":"stu_1"},{"Id":2,"Gender":"男","Name":"stu_2"},{"Id":3,"Gender":"男","Name":"stu_3"},{"Id":4,"Gender":"男","Name":"stu_4"},{"Id":5,"Gender":"男","Name":"stu_5"},{"Id":6,"Gender":"男","Name":"stu_6"},{"Id":7,"Gender":"男","Name":"stu_7"},{"Id":8,"Gender":"男","Name":"stu_8"},{"Id":9,"Gender":"男","Name":"stu_9"},{"Id":10,"Gender":"男","Name":"stu_10"}]}`
var c = &Class{}
err := json.Unmarshal([]byte(str),c)
if err != nil {
fmt.Println(err)
} else {
fmt.Printf("%#v\n", c)
fmt.Printf("%v", c.Title)
}
// &main.Class{Title:"01班", Students:[]main.Student{main.Student{Id:1, Gender:"男", Name:"stu_1"}, main.Student{Id:2, Gender:"男", Name:"stu_2"}, main.Student{Id:3, Gender:"男", Name:"stu_3"}, main.Student{Id:4, Gender:"男", Name:"stu_4"}, main.Student{Id:5, Gender:"男", Name:"stu_5"}, main.Student{Id:6, Gender:"男", Name:"stu_6"}, main.Student{Id:7, Gender:"男", Name:"stu_7"}, main.Student{Id:8, Gender:"男", Name:"stu_8"}, main.Student{Id:9, Gender:"男", Name:"stu_9"}, main.Student{Id:10, Gender:"男", Name:"stu_10"}}}
//01班
}
结构体标签Tag
通过指定tag实现json序列化该字段时的key
type Student struct {
Id int `json:"id"`
Gender string `json:"gender"`
Name string `json:"name"`
Sno string `json:"no"`
}
func main (){
var s1 = Student {
Id : 12,
Gender :"男",
Name : "李四",
Sno : "s001",
}
jsonByte, _ := json.Marshal(s1)
jsonStr := string(jsonByte)
fmt.Printf("%v", jsonStr)
//{"id":12,"gender":"男","name":"李四","no":"s001"}
}
本文来自:简书
感谢作者:learninginto
查看原文:18 Golang结构体详解(四)
相关阅读 >>
介绍基于Go语言框架gin开发的mvc轮子框架:ginlaravel
Google官宣:新一代操作系统fuchsia编程语言竟然是它!
更多相关阅读请进入《Go》频道 >>
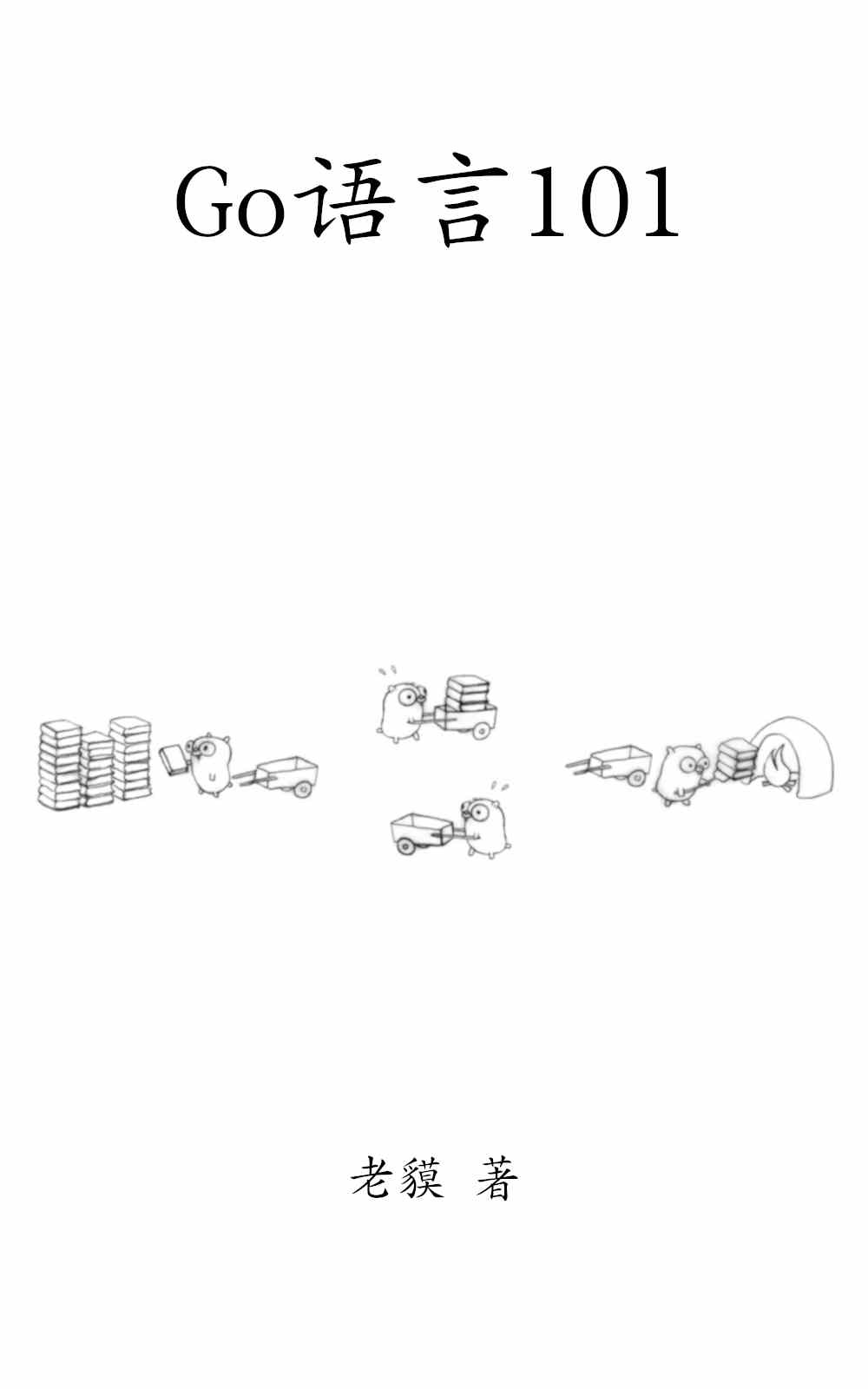
Go语言101
一个与时俱进的Go编程知识库。