本文摘自网络,作者,侵删。
封装
封装主要是通过访问权限控制实现的。
在Java中,共有public 、protected、default、private这四种权限控制。
而相应的在golang中,是通过约定来实现权限控制的。变量名首字母大写,相当于java中的public,首字母小写,相当于private。同一个包中访问,相当于default。由于go没有继承,也就没有protected。
继承
虽然golang的语法没有继承,但是可以通过相应的结构体之间的组合来实现类似的继承效果。
例子如下:
package main
import "fmt"
type inner struct {
i string
}
func (in *inner) innerMethod() {
fmt.Println("inner's innerMethod!!!")
}
type outter struct {
s1 string
s2 int
s3 bool
inner
}
func main() {
o := new(outter)
fmt.Printf("o.i %v\n", o.i)
o.i = "inner"
fmt.Printf("o.i %v\n", o.i)
o.innerMethod()
}
输出结果
o.i
o.i inner
inner's innerMethod!!!
也可以重写innerMethod方法,例子如下:
package main
import "fmt"
type inner struct {
i string
}
func (in *inner) innerMethod() {
fmt.Println("inner's innerMethod!!!")
}
type outter struct {
s1 string
s2 int
s3 bool
inner
}
func (o *outter) innerMethod(s string) {
fmt.Println("outter's innerMethod!!!")
}
func main() {
o := new(outter)
fmt.Printf("o.i %v\n", o.i)
o.i = "inner"
fmt.Printf("o.i %v\n", o.i)
//o.innerMethod()
o.innerMethod("test")
}
输出结果:
o.i
o.i inner
outter's innerMethod!!!
多态
golang中,只要某个struct实现了某个interface中的所有方法,那么我们就认为,这个struct实现了这个类。
例子如下:
package main
import "fmt"
type Person interface {
Action()
}
type Girl struct {
Name string
}
func (g *Girl) Action() {
fmt.Printf("My name is %v\n", g.Name)
}
type Boy struct {
Name string
}
func (b *Boy) Action() {
fmt.Printf("My name is %v\n", b.Name)
}
func main() {
girl := &Girl{Name: "Beautiful"}
boy := &Boy{Name: "Handsome"}
girl.Action()
boy.Action()
}
输出结果:
My name is Beautiful
My name is Handsome
本文来自:简书
感谢作者:陈光环_18
查看原文:Go封装、继承、多态
相关阅读 >>
知乎从python转为Go,是不是代表Go比python好?
海康/大华sdk协议easycvr如何通过Go语言读取csv文件内容?
更多相关阅读请进入《Go》频道 >>
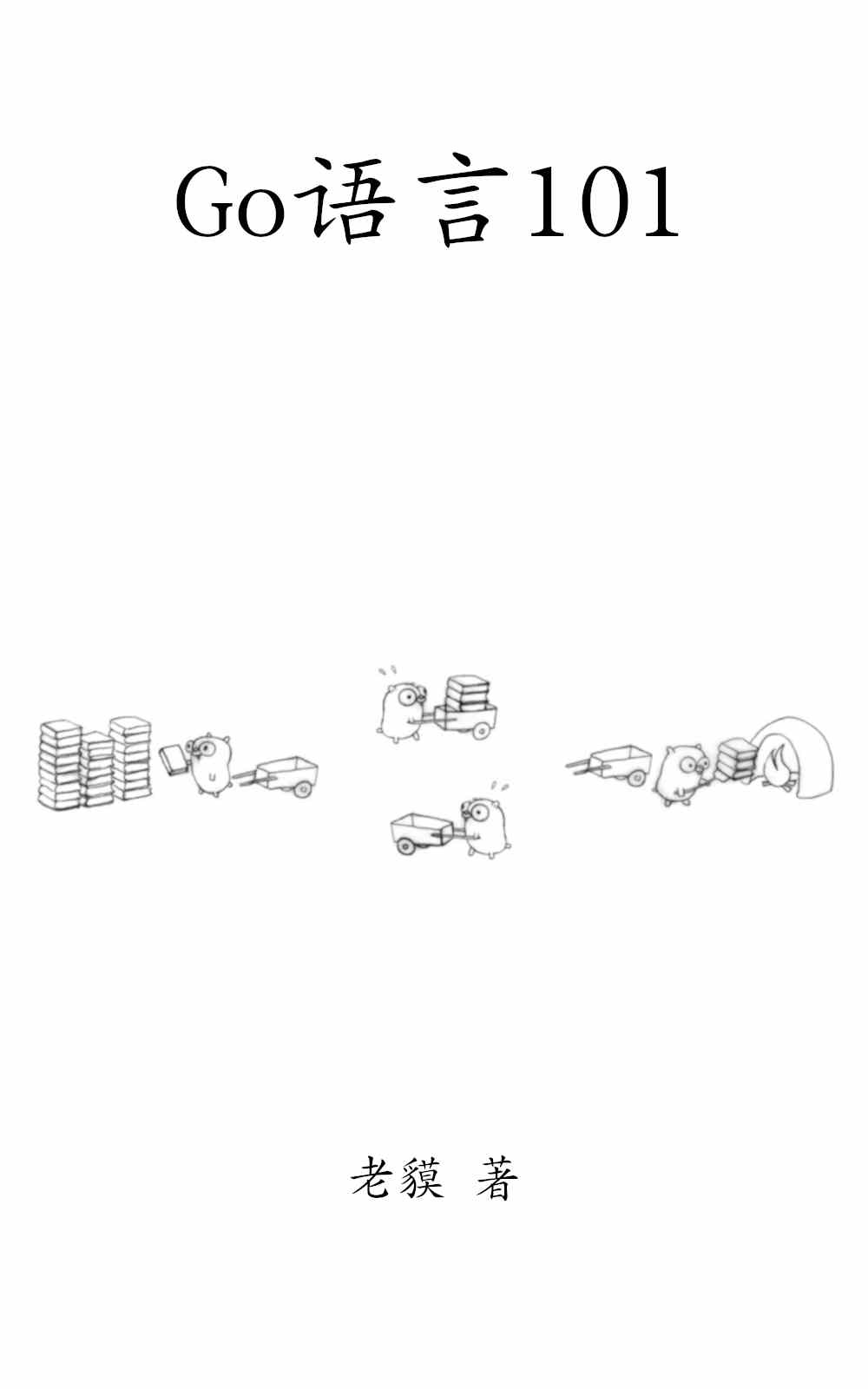
Go语言101
一个与时俱进的Go编程知识库。