本文摘自网络,作者,侵删。
根据GO夜读学习go源码
源码:
// The make built-in function allocates and initializes an object of type
// slice, map, or chan (only). Like new, the first argument is a type, not a
// value. Unlike new, make's return type is the same as the type of its
// argument, not a pointer to it. The specification of the result depends on
// the type:
// Slice: The size specifies the length. The capacity of the slice is
// equal to its length. A second integer argument may be provided to
// specify a different capacity; it must be no smaller than the
// length. For example, make([]int, 0, 10) allocates an underlying array
// of size 10 and returns a slice of length 0 and capacity 10 that is
// backed by this underlying array.
// Map: An empty map is allocated with enough space to hold the
// specified number of elements. The size may be omitted, in which case
// a small starting size is allocated.
// Channel: The channel's buffer is initialized with the specified
// buffer capacity. If zero, or the size is omitted, the channel is
// unbuffered.
// make内置函数用于分配和初始化slice、map或chan(仅限于)类型的对象。与new一样,第一个参数是类型,而不是值。与new不同,make的返回类型与其参数的类型相同,而不是指向它的指针。返回结果的规范取决于传入的类型:
// 切片:大小指定长度。切片的容量等于其长度。可以提供第二个整数参数来指定不同的容量;它不能小于长度。例如,make([]int,0,10)分配一个大小为10的底层数组,并返回一个长度为0、容量为10的切片,该切片由这个底层数组支持。
// 映射:为空映射分配足够的空间来容纳指定数量的元素。可以省略大小,在这种情况下,分配小的起始大小。
// 通道:使用指定的缓冲区容量初始化通道的缓冲区。如果为零,或者忽略了大小,则通道是无缓冲的。
func make(t Type, size ...IntegerType) Type
// The new built-in function allocates memory. The first argument is a type,
// not a value, and the value returned is a pointer to a newly
// allocated zero value of that type.
// new内置函数分配内存。第一个参数是类型,而不是值,返回的值是指向该类型的新分配的零值的指针。
func new(Type) *Type
//
Demo
package main
import (
"fmt"
)
func main() {
p := new([]int)
fmt.Println(*p) // p !=nil ,指向该类型的新分配的零值的指针。
t := make([]int, 0, 10) // t !=nil
// new([]int)和make([]int, 0)有什么区别?
// 如果make第二个参数(容量)不是0的话,会初始化对应的默认零值填充数组。
// 而new初始化的对象也可以指定容量比如 new([10]int)和make([]int,10)初始化的容量相同,但是new是返回的是指针而make不是!!!
fmt.Println(t)
}
Output
// output
// 可以很明显的看出new函数返回的是一个指针,类型是传递的类型
&[]
// make 返回的是一个类型对象,而不是像new一样返回一个指针
[0 0 0 0 0 0 0 0 0 0]
Append
Demo
在往slice里append元素是可以发现其有不同的地方,看下面代码
package main
import "fmt"
func main() {
p := make([]int, 0,10)
p = append(p, 1)
fmt.Println(p)
t := make([]int, 10, 10)
t = append(t, 1)
fmt.Println(t)
}
Output
// output
[1]
[0 0 0 0 0 0 0 0 0 0 1]
咦,这个时候不知道的同学可能会问,同样是追加为什么两个输出不一样呢?
注意看第5行代码,初始话的是容量为0的Slice,append函数是在数据的最后面追加元素,我们看下源码:
源码
// The append built-in function appends elements to the end of a slice. If
// it has sufficient capacity, the destination is resliced to accommodate the
// new elements. If it does not, a new underlying array will be allocated.
// Append returns the updated slice. It is therefore necessary to store the
// result of append, often in the variable holding the slice itself:
// slice = append(slice, elem1, elem2)
// slice = append(slice, anotherSlice...)
// As a special case, it is legal to append a string to a byte slice, like this:
// slice = append([]byte("hello "), "world"...)
// append内置函数将元素追加到切片的末尾。 如果具有足够的容量,则将目标切片为容纳新元素。 如果没有,将分配一个新的底层数组。
// 追加返回更新的切片。 因此,有必要将添加的结果存储在通常包含切片本身的变量中:
// slice =append(slice,elem1,elem2)
// slice = append(slice,anotherSlice ...)
// 作为一种特殊情况,将字符串附加到字节片是合法的,如下所示:
// slice = append([] byte("hello"), "world" ...)
func append(slice []Type, elems ...Type) []Type
也就是说当make中第二个参数(第二个参数比作初始化的小,把第三个参数比做数据的容量),容量指的是当前切片可以容纳多少个元素不用扩容,长度是指当前切片有多个元素。比如make([]int,10,10)
也就是说当前切片容量为50个元素,一共有10个默认值被初始化零值,所以说长度小于容量时是不会发生扩容的。
如果append元素当前长度不大于当前容量,是不会创建新的切片的,咱们可以看下内存地址。
package main
import "fmt"
func main() {
t := make([]int, 1,10)
fmt.Println(t)
fmt.Println(&t[0])
t = append(t, 1)
fmt.Println(t)
fmt.Println(&t[0])
//case 2 追加超过容量
p := make([]int, 10, 10)
fmt.Println(p)
fmt.Println(&p[0])
p = append(p, 1)
fmt.Println(p)
fmt.Println(&p[0])
}
// output
[0]
0xc00009c050
[0 1]
0xc00009c050
// case2 output
[0 0 0 0 0 0 0 0 0 0]
0xc00001e050
[0 0 0 0 0 0 0 0 0 0 1]
0xc0000140a0
通过以上测试可以知道append函数是往当前切片长度最后追加,如果追加后长度超过了容量将分配一个新的底层数组。
本文来自:简书
感谢作者:Eizeson
查看原文:【Golang】make和new区别,append
相关阅读 >>
更多相关阅读请进入《Go》频道 >>
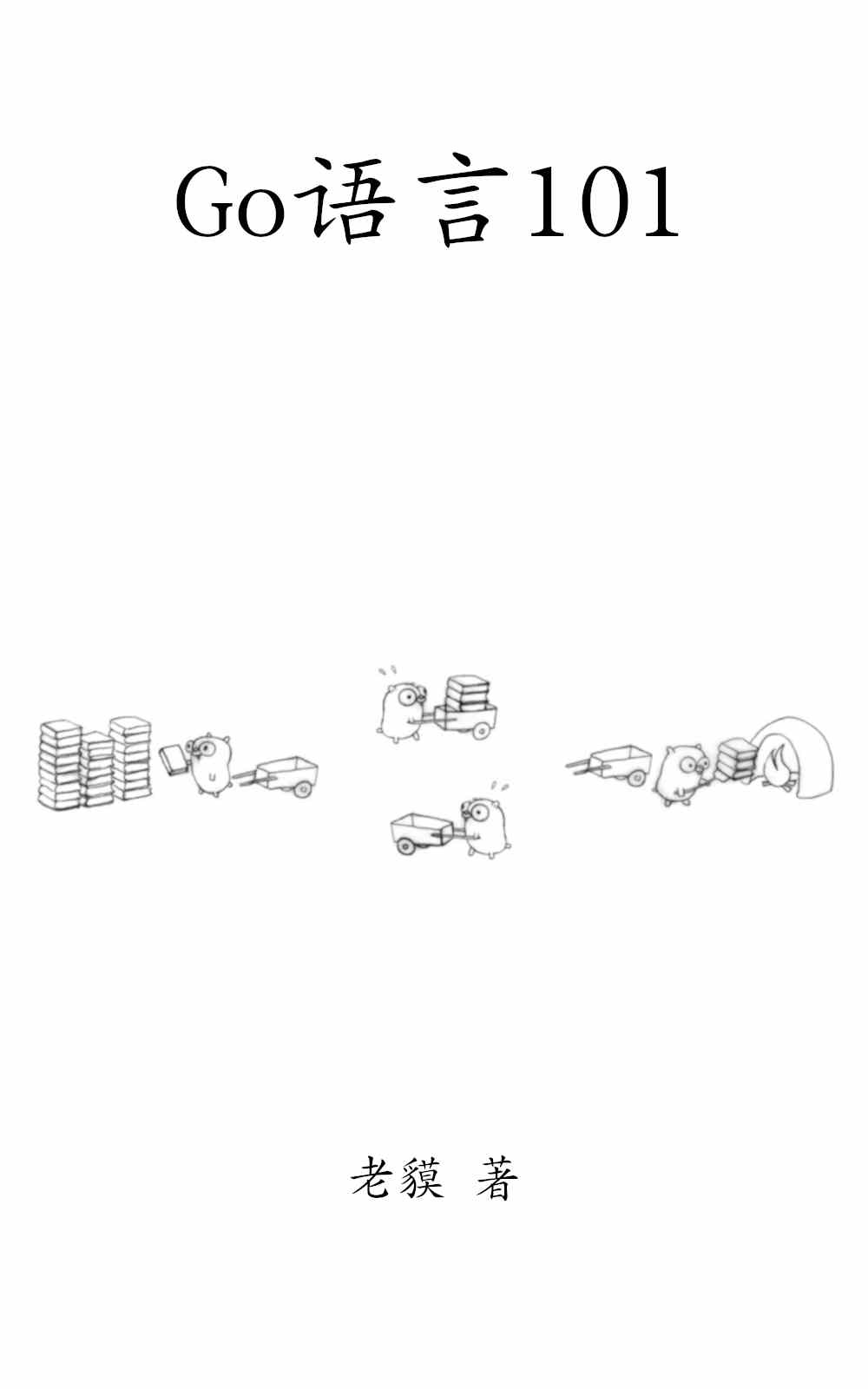
Go语言101
一个与时俱进的Go编程知识库。