本文摘自网络,作者,侵删。
title: "Golang Array Slice操作示例 去重 插入 删除 清空"
date: 2021-02-16T21:21:32+08:00
draft: true
tags: ['go']
author: "dadigang"
author_cn: "大地缸"
personal: "http://www.real007.cn"
关于作者
http://www.real007.cn/about
[Golang]Slice操作示例(去重、插入、删除、清空)
1. Slice去重操作:
/* 在slice中去除重复的元素,其中a必须是已经排序的序列。
* params:
* a: slice对象,如[]string, []int, []float64, ...
* return:
* []interface{}: 已经去除重复元素的新的slice对象
*/
func SliceRemoveDuplicate(a interface{}) (ret []interface{}) {
if reflect.TypeOf(a).Kind() != reflect.Slice {
fmt.Printf("<SliceRemoveDuplicate> <a> is not slice but %T\n", a)
return ret
}
va := reflect.ValueOf(a)
for i := 0; i < va.Len(); i++ {
if i > 0 && reflect.DeepEqual(va.Index(i-1).Interface(), va.Index(i).Interface()) {
continue
}
ret = append(ret, va.Index(i).Interface())
}
return ret
}
运行测试代码:
func test_SliceRemoveDuplicate() {
slice_string := []string{"a", "b", "c", "d", "a", "b", "c", "d"}
slice_int := []int{1, 2, 3, 4, 5, 1, 2, 3, 4, 5}
slice_float := []float64{1.11, 2.22, 3.33, 4.44, 1.11, 2.22, 3.33, 4.44}
sort.Strings(slice_string)
sort.Ints(slice_int)
sort.Float64s(slice_float)
fmt.Printf("slice_string = %v, %p\n", slice_string, slice_string)
fmt.Printf("slice_int = %v, %p\n", slice_int, slice_int)
fmt.Printf("slice_float = %v, %p\n", slice_float, slice_float)
ret_slice_string := SliceRemoveDuplicate(slice_string)
ret_slice_int := SliceRemoveDuplicate(slice_int)
ret_slice_float := SliceRemoveDuplicate(slice_float)
fmt.Printf("ret_slice_string = %v, %p\n", ret_slice_string, ret_slice_string)
fmt.Printf("ret_slice_int = %v, %p\n", ret_slice_int, ret_slice_int)
fmt.Printf("ret_slice_float = %v, %p\n", ret_slice_float, ret_slice_float)
fmt.Printf("<after> slice_string = %v, %p\n", slice_string, slice_string)
fmt.Printf("<after> slice_int = %v, %p\n", slice_int, slice_int)
fmt.Printf("<after> slice_float = %v, %p\n", slice_float, slice_float)
}
输出结果如下:
slice_string = [a a b b c c d d], 0xc042088000
slice_int = [1 1 2 2 3 3 4 4 5 5], 0xc04200e1e0
slice_float = [1.11 1.11 2.22 2.22 3.33 3.33 4.44 4.44], 0xc042014200
ret_slice_string = [a b c d], 0xc042034100
ret_slice_int = [1 2 3 4 5], 0xc042088080
ret_slice_float = [1.11 2.22 3.33 4.44], 0xc042034180
<after> slice_string = [a a b b c c d d], 0xc042088000
<after> slice_int = [1 1 2 2 3 3 4 4 5 5], 0xc04200e1e0
<after> slice_float = [1.11 1.11 2.22 2.22 3.33 3.33 4.44 4.44], 0xc042014200
2. Slice插入操作:
/*
* 在Slice指定位置插入元素。
* params:
* s: slice对象,类型为[]interface{}
* index: 要插入元素的位置索引
* value: 要插入的元素
* return:
* 已经插入元素的slice,类型为[]interface{}
*/
func SliceInsert(s []interface{}, index int, value interface{}) []interface{} {
rear := append([]interface{}{}, s[index:]...)
return append(append(s[:index], value), rear...)
}
/*
* 在Slice指定位置插入元素。
* params:
* s: slice对象指针,类型为*[]interface{}
* index: 要插入元素的位置索引
* value: 要插入的元素
* return:
* 无
*/
func SliceInsert2(s *[]interface{}, index int, value interface{}) {
rear := append([]interface{}{}, (*s)[index:]...)
*s = append(append((*s)[:index], value), rear...)
}
/*
* 在Slice指定位置插入元素。
* params:
* s: slice对象的指针,如*[]string, *[]int, ...
* index: 要插入元素的位置索引
* value: 要插入的元素
* return:
* true: 插入成功
* false: 插入失败(不支持的数据类型)
*/
func SliceInsert3(s interface{}, index int, value interface{}) bool {
if ps, ok := s.(*[]string); ok {
if val, ok := value.(string); ok {
rear := append([]string{}, (*ps)[index:]...)
*ps = append(append((*ps)[:index], val), rear...)
return true
}
} else if ps, ok := s.(*[]int); ok {
if val, ok := value.(int); ok {
rear := append([]int{}, (*ps)[index:]...)
*ps = append(append((*ps)[:index], val), rear...)
}
} else if ps, ok := s.(*[]float64); ok {
if val, ok := value.(float64); ok {
rear := append([]float64{}, (*ps)[index:]...)
*ps = append(append((*ps)[:index], val), rear...)
}
} else {
fmt.Printf("<SliceInsert3> Unsupported type: %T\n", s)
}
return false
}
说明:
1. SliceInsert()方法是传入一个[]interface{}类型的slice对象,返回的也是一个[]interface{}类型的slice对象。
2. SliceInsert2()方法是传入一个[]interface{}类型的slice对象指针,直接修改这个slice对象。
3. SliceInsert3()方法是传入一个具体类型的slice对象指针(如*[]string, *[]int等),方法中直接修改这个slice对象,返回操作是否成功的状态(bool)。
相关阅读 >>
聊聊dubbo-Go-proxy的zookeeperregistryload
更多相关阅读请进入《Go》频道 >>
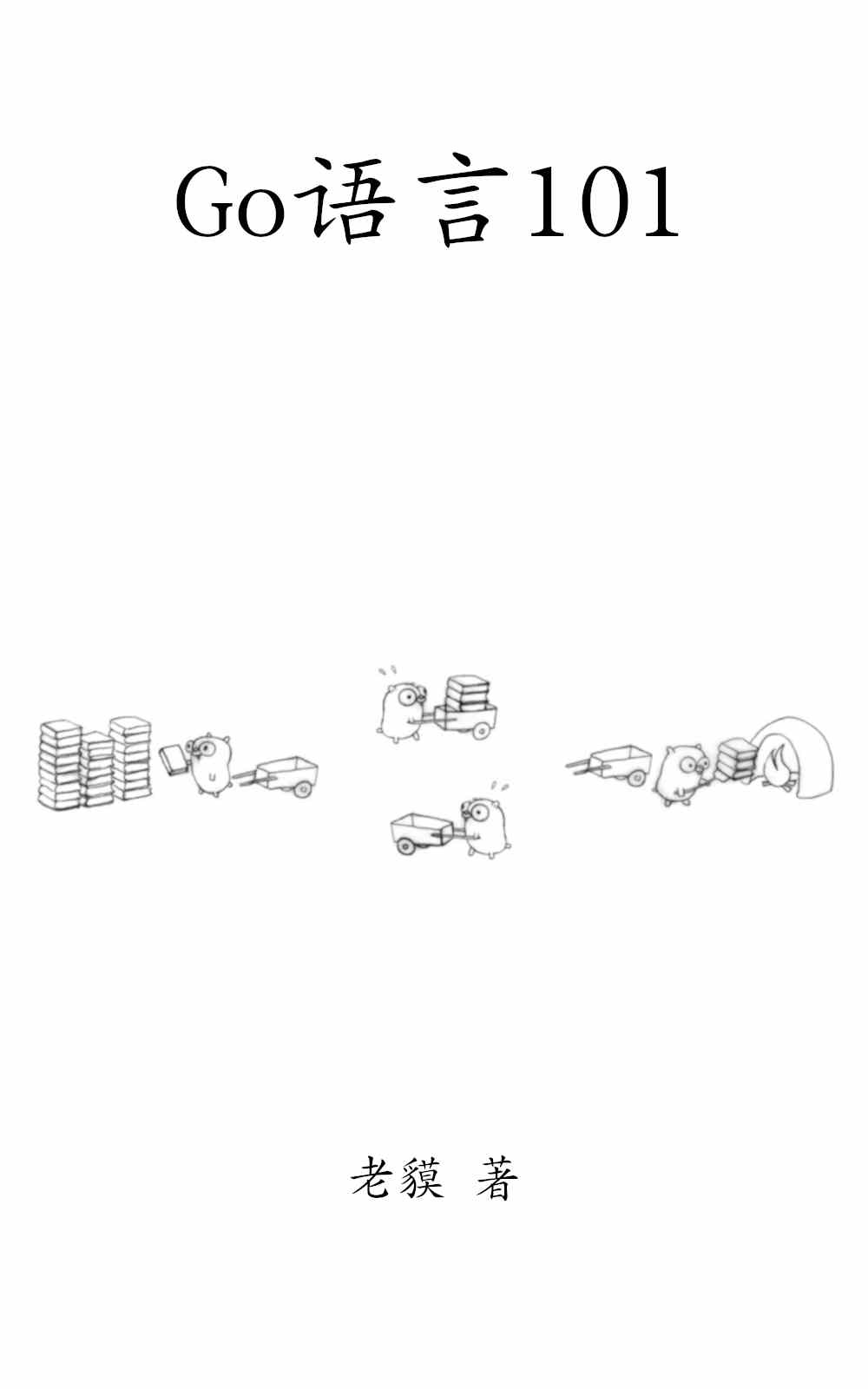
Go语言101
一个与时俱进的Go编程知识库。