本文摘自网络,作者,侵删。
接口
定义及使用
package main
import (
"fmt"
)
//定义一个接口
type Human interface {
sayHello()
}
type Student struct {
name string
age int
}
type Teacher struct {
group string
}
func (stu *Student) sayHello() {
fmt.Printf("Student[%s,%d] \n", stu.name, stu.age)
}
func (tea *Teacher) sayHello() {
fmt.Printf("Teacher[%s] \n", tea.group)
}
func WhoSay(tmp Human) {
tmp.sayHello()
}
func main() {
//第一种使用
s := &Student{"Bon", 12}
t := &Teacher{"math"}
WhoSay(s)
WhoSay(t)
//第二种使用 创建切片
slic := make([]Human, 2)
slic[0] = s
slic[1] = t
for _, v := range slic {
v.sayHello()
}
}
接口继承
package main
import (
"fmt"
)
//定义一个接口
type Humaner interface {
sayHello()
}
//继承Humaner
type Personer interface {
Humaner
sayWorld(str string)
}
type Student struct {
name string
age int
}
func (stu *Student) sayHello() {
fmt.Printf("Student[%s,%d] \n", stu.name, stu.age)
}
func (stu *Student) sayWorld(str string) {
fmt.Println(str)
}
func main() {
var i Personer
s := &Student{"Bob", 23}
i = s
i.sayHello()
i.sayWorld("song")
}
接口转化(超集可以转化为子集,子集不可以转化为超集)
package main
import (
"fmt"
)
//定义一个接口
type Humaner interface { //子集
sayHello()
}
type Personer interface { //超集
Humaner
sayWorld(str string)
}
type Student struct {
name string
age int
}
func (stu *Student) sayHello() {
fmt.Printf("Student[%s,%d] \n", stu.name, stu.age)
}
func (stu *Student) sayWorld(str string) {
fmt.Println(str)
}
func main() {
var i Personer
i = &Student{"Tom", 16}
var h Humaner
//子集不能转为超集
// i = h
//超集可以转化为子集
h = i
h.sayHello()
}
空接口(可以保存任意类型的数据)
package main
import (
"fmt"
)
func xxx(arg ...interface{}) {
}
func main() {
//空接口可保存任意类型的数据
var i interface{}
i = 10
fmt.Println(i)
var m interface{}
m = "hello world"
fmt.Println(m)
}
判断空接口存储的数据类型
package main
import (
"fmt"
)
func main() {
var i interface{}
i = 10
//使用 变量.(类型) 判断数据数据类型
if value, ok := i.(int); ok == true {
fmt.Println(value)
}
}
package main
import (
"fmt"
)
func main() {
var i interface{}
i = 10
//使用 变量.(type) 判断数据数据类型
switch value := i.(type) {
case int:
fmt.Printf("int 类型 %d \n", value)
case string:
fmt.Print("string 类型 %s \n", value)
}
}
本文来自:51CTO博客
感谢作者:mb601cf691d1fe2
查看原文:golang学习笔记——面向对象(接口)
相关阅读 >>
beeGo-vue-admin基于当前流行技术组合的前后端rbac管理系统:Go1.15.x+beeGo2.x+jwt+redis+mysql8+vue 的前后端分离系统,权限控制采用 rbac,支持
更多相关阅读请进入《Go》频道 >>
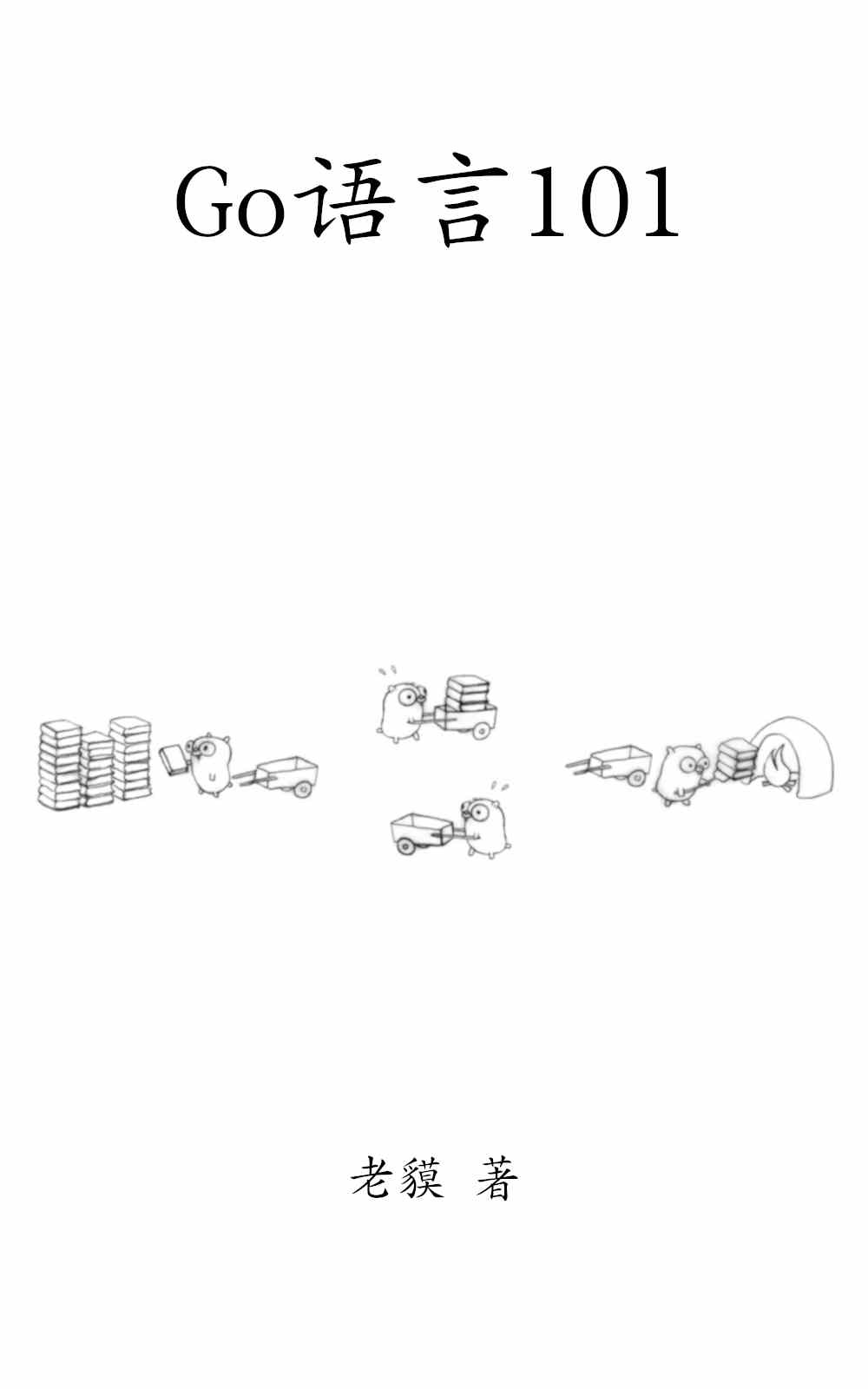
Go语言101
一个与时俱进的Go编程知识库。