本文摘自网络,作者,侵删。
package main
import (
"errors"
"fmt"
"os"
)
type Queue struct {
MaxSize int
Array []int
Front int
Rear int
}
func (this *Queue) AddQueue(val int) error {
// 判断队列是否已满
if this.Rear-this.Front >= this.MaxSize {
return errors.New("queue full")
}
// 数组偏移下标
this.Rear++
this.Array[this.Rear%this.MaxSize] = val
return nil
}
func (this *Queue) GetQueue() (int, error) {
if this.Rear == this.Front {
return -1, errors.New("queue empty")
}
this.Front++
return this.Array[this.Front%this.MaxSize], nil
}
func (this *Queue) ShowQueue() {
fmt.Print("queue:")
for i := this.Front + 1; i <= this.Rear; i++ {
fmt.Printf("\tarr[%d]=%d", i%this.MaxSize, this.Array[i%this.MaxSize])
}
fmt.Println()
}
func main() {
queue := &Queue{MaxSize: 5, Front: -1, Rear: -1, Array: make([]int, 5)}
var action string
var val int
for {
fmt.Println("1.输入add 表示添加数据到队列\n2.输入get 表示从队列获取数据\n3.输入show 表示显示队列\n4.输入exit 退出程序")
fmt.Scanln(&action)
switch action {
case "add":
fmt.Println("输入要入队列数:")
fmt.Scanln(&val)
err := queue.AddQueue(val)
if err == nil {
fmt.Printf("\t加入队列成功\n")
} else {
fmt.Printf("\t%s\n", err.Error())
}
case "get":
val, err := queue.GetQueue()
if err == nil {
fmt.Printf("\t从队列中取出了: %d\n", val)
} else {
fmt.Printf("\t%s\n", err.Error())
}
case "show":
queue.ShowQueue()
case "exit":
os.Exit(0)
}
}
}
本文来自:简书
感谢作者:_H_8f4a
查看原文:Golang实现数组模拟环形队列
相关阅读 >>
聊聊dubbo-Go-proxy的consulregistryload
更多相关阅读请进入《Go》频道 >>
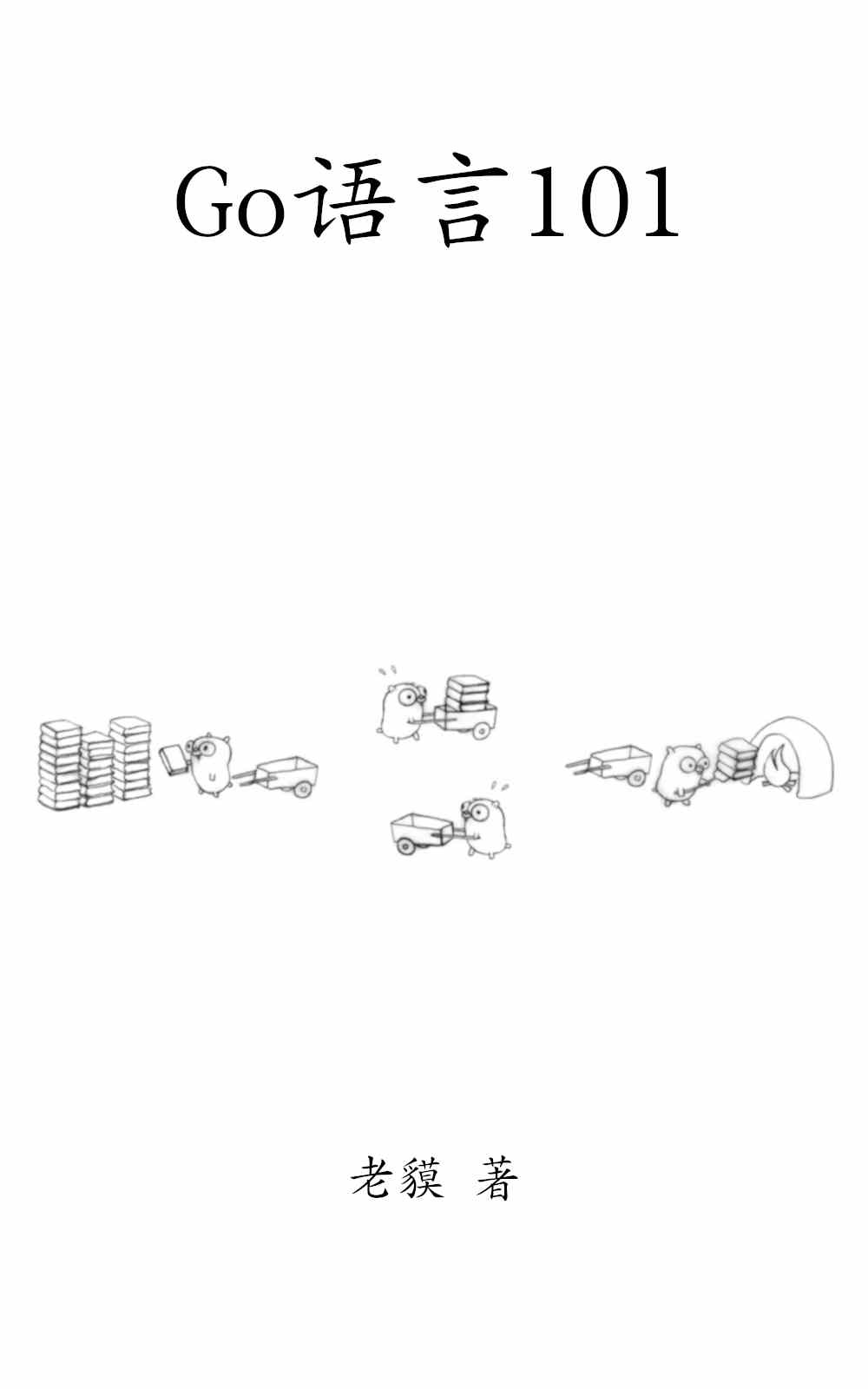
Go语言101
一个与时俱进的Go编程知识库。