本文摘自网络,作者,侵删。
title: "Golang连接elasticsearch"
date: 2021-02-10T21:41:32+08:00
draft: true
tags: ['go','elasticsearch']
author: "dadigang"
author_cn: "大地缸"
personal: "http://www.real007.cn"
关于作者
http://www.real007.cn/about
golang连接elasticsearch
package main
import (
"github.com/olivere/elastic"
"fmt"
"context"
)
type Tweet struct {
User string
Message string
Retweets int64
}
func main() {
client, err := elastic.NewClient(elastic.SetURL("http://192.168.33.134:9200"))
if err != nil {
// Handle error
}
fmt.Println(client)
// Use the IndexExists service to check if a specified index exists.
exists, err := client.IndexExists("twitter").Do(context.Background())
if err != nil {
// Handle error
panic(err)
}
if !exists {
// Create a new index.
mapping := `
{
"settings":{
"number_of_shards":1,
"number_of_replicas":0
},
"mappings":{
"doc":{
"properties":{
"user":{
"type":"keyword"
},
"message":{
"type":"text",
"store": true,
"fielddata": true
},
"retweets":{
"type":"long"
},
"tags":{
"type":"keyword"
},
"location":{
"type":"geo_point"
},
"suggest_field":{
"type":"completion"
}
}
}
}
}
`
createIndex, err := client.CreateIndex("twitter").Body(mapping).Do(context.Background())
if err != nil {
// Handle error
panic(err)
}
if !createIndex.Acknowledged {
// Not acknowledged
}
}
// Index a tweet (using JSON serialization)
tweet1 := Tweet{User: "olivere", Message: "Take Five", Retweets: 0}
put1, err := client.Index().
Index("twitter").
Type("doc").
Id("1").
BodyJson(tweet1).
Do(context.Background())
if err != nil {
// Handle error
panic(err)
}
fmt.Printf("Indexed tweet %s to index %s, type %s\n", put1.Id, put1.Index, put1.Type)
// Index a second tweet (by string)
tweet2 := `{"user" : "olivere", "message" : "It's a Raggy Waltz"}`
put2, err := client.Index().
Index("twitter").
Type("doc").
Id("2").
BodyString(tweet2).
Do(context.Background())
if err != nil {
// Handle error
panic(err)
}
fmt.Printf("Indexed tweet %s to index %s, type %s\n", put2.Id, put2.Index, put2.Type)
// Get tweet with specified ID
get1, err := client.Get().
Index("twitter").
Type("doc").
Id("1").
Do(context.Background())
fmt.Println(get1)
if err != nil {
switch {
case elastic.IsNotFound(err):
panic(fmt.Sprintf("Document not found: %v", err))
case elastic.IsTimeout(err):
panic(fmt.Sprintf("Timeout retrieving document: %v", err))
case elastic.IsConnErr(err):
panic(fmt.Sprintf("Connection problem: %v", err))
default:
// Some other kind of error
panic(err)
}
}
}
本文来自:简书
感谢作者:大地缸
查看原文:Golang连接elasticsearch
相关阅读 >>
Go - 基于 Gorm 获取当前请求所执行的 sql 信息
更多相关阅读请进入《Go》频道 >>
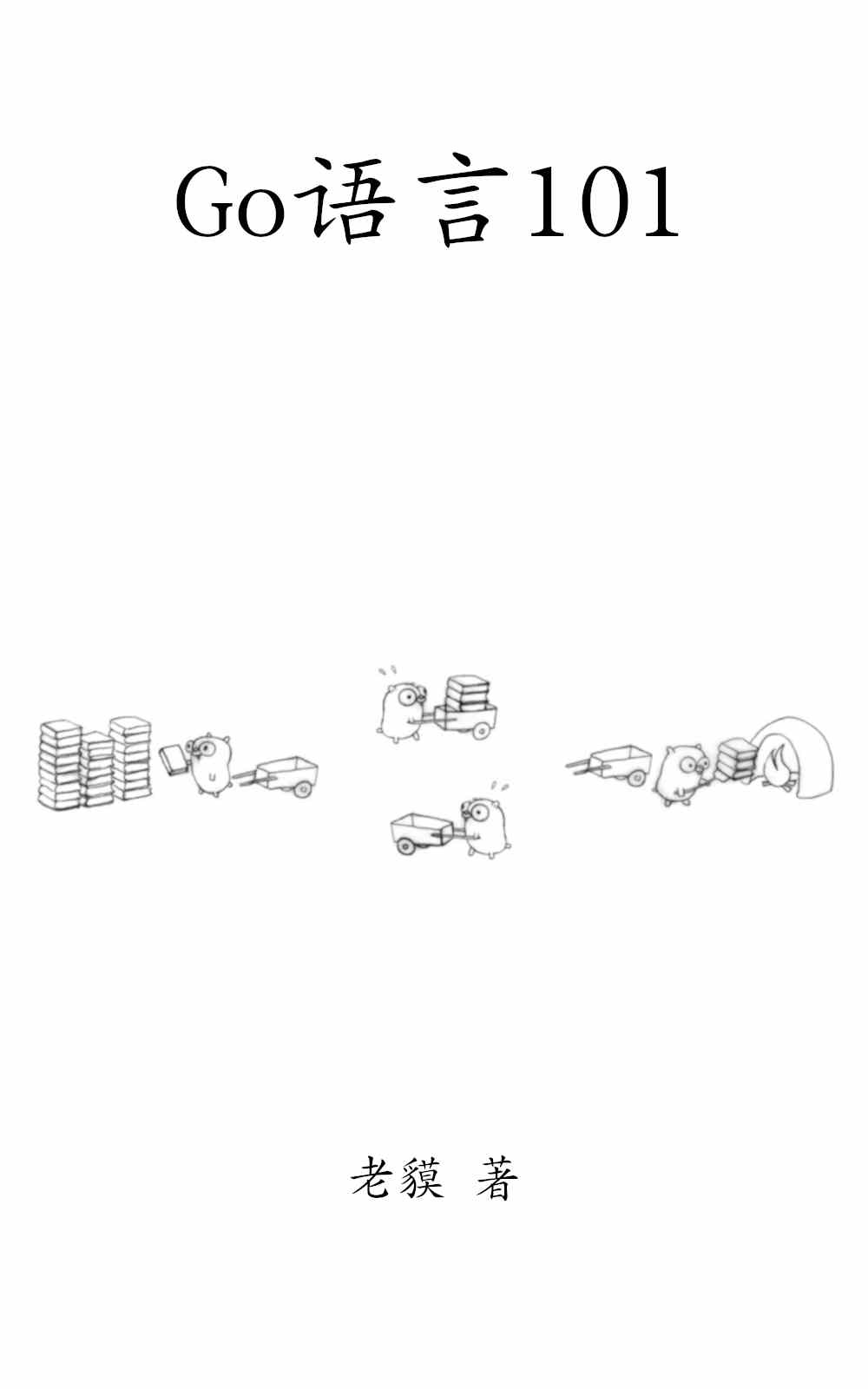
Go语言101
一个与时俱进的Go编程知识库。