本文摘自PHP中文网,作者小葫芦,侵删。
若要了解有关 Visual Studio 2017 RC 的最新文档,请参阅 Visual Studio 2017 RC 文档。
在通过 dynamic 类型实现的操作中,该类型的作用是绕过编译时类型检查, 改为在运行时解析这些操作。 dynamic 类型简化了对 COM API(例如 Office Automation API)、动态 API(例如 IronPython 库)和 HTML 文档对象模型 (DOM) 的访问。
在大多数情况下,dynamic 类型与 object 类型的行为是一样的。 但是,不会用编译器对包含 dynamic 类型表达式的操作进行解析或类型检查。 编译器将有关该操作信息打包在一起,并且该信息以后用于计算运行时操作。 在此过程中,类型 dynamic 的变量会编译到类型 object 的变量中。 因此,类型 dynamic 只在编译时存在,在运行时则不存在。
以下示例将类型为 dynamic 的变量与类型为 object 的变量对比。 若要在编译时验证每个变量的类型,请将鼠标指针放在 WriteLine 语句中的 dyn或 obj 上。 IntelliSense 显示了 dyn 的“动态”和 obj 的“对象”。
1 2 3 4 5 6 7 8 |
|
WriteLine 语句显示 dyn 和 obj 的运行时类型。 此时,两者具有相同的整数类型。 将生成以下输出:
System.Int32
System.Int32
若要查看 dyn 和 obj 之间的差异,请在前面示例的声明和 WriteLine 语句之间添加下列两行之间。
1 2 |
|
为尝试添加表达式 obj + 3 中的整数和对象报告编译器错误。 但是,不会报告 dyn + 3 错误。 编译时不会检查包含 dyn 的表达式,原因是 dyn 的类型为 dynamic。
上下文
dynamic 关键字可以直接出现或作为构造类型的组件在下列情况中出现:
在声明中,作为属性、字段、索引器、参数、返回值或类型约束的类型。 下面的类定义在几个不同的声明中使用 dynamic。
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
在显式类型转换中,作为转换的目标类型。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
在以类型充当值(如 is 运算符或 as 运算符右侧)或者作为 typeof 的参数成为构造类型的一部分的任何上下文中。 例如,可以在下列表达式中使用 dynamic。
1 2 3 4 5 6 7 |
|
示例
下面的示例以多个声明使用 dynamic。 Main 也用运行时类型检查对比编译时类型检查。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
相关阅读 >>
c# webservice中访问http和https的wsdl以及生成配置节点的不同之处 (图)
c# invoke 和 begininvoke之间的区别详解
.net core中如何使用entity framework操作postgresql?
详细分析.net?core?以及与?.net?framework的关系(图)
更多相关阅读请进入《csharp》频道 >>
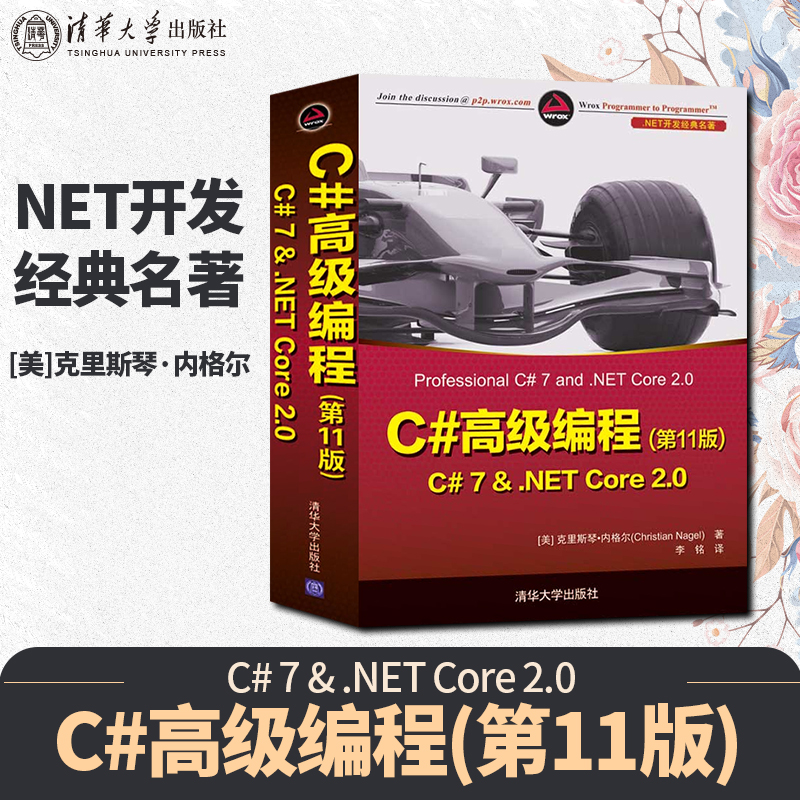
C#高级编程(第11版) C# 7 & .NET Core 2.0(.NET开发经典名著)
作者:[美]克里斯琴·内格尔(Christian Nagel)著。出版时间:2019年3月。