本文整理自网络,侵删。
在pom.xml添加相应的依赖
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.3</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 前端使用thymeleaf来代替jsp --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies>
配置文件配置数据库等
#server server.port=80 #项目名:server.servlet.context-path #spring dataSource spring.datasource.url=jdbc:mysql:///dbgoods?serverTimezone=GMT%2B8&characterEncoding=utf8 spring.datasource.username=root spring.datasource.password=root mybatis.mapper-locations=classpath:/mapper/*/*.xml #spring log logging.level.com.cy=debug #spring thymeleaf(假如没有配置也会默认配置,在默认配置中prefix默认值为classpath:/templates/,后缀默认为.html) #不用重启服务器,网页就能刷新 spring.thymeleaf.cache=false spring.thymeleaf.prefix=classpath:/templates/pages/ spring.thymeleaf.suffix=.html
数据层添加相应注解实现sql语句(或者通过xml配置来实现)
数据层封装了商品信息,并提供get和set方法,为Goods类
1.查询所有数据
@Select("select * from tb_goods") List<Goods> findAll();
2.按照id删除数据
@Delete("delete from tb_goods where id=#{id}") int deleteById(Integer id);
3.修改数据
(1)修改数据首先要新建一个界面,按照id查找内容,并将查找到的内容显示到文本框内
@Select("select * from tb_goods where id=#{id}") Goods findById(Integer id);
(2)再添加查找的方法
@Update("update tb_goods set name=#{name},remark=# {remark},createdTime=now() where id=#{id}") int update(Goods goods);
4.新增数据
@Insert("insert into tb_goods(name,remark,createdTime) values (#{name},#{remark},now())") int add(Goods goods);
业务层提供对应接口方法和实现类
1.业务层接口
public interface GoodsService { List<Goods> findObject(); int add(Goods goods); int update(Goods goods); Goods findById(Integer id); }
2.业务层实现类
@Service public class GoodsServiceImpl implements GoodsService { @Autowired private GoodsDao goodsDao; @Override public List<Goods> findObject() { long start=System.currentTimeMillis(); List<Goods> list = goodsDao.findObjects(); long end=System.currentTimeMillis(); System.out.println("query time:"+(end-start)); return list; } @Override public int add(Goods goods) { return goodsDao.add(goods); } @Override public int update(Goods goods) { return goodsDao.update(goods); } @Override public Goods findById(Integer id) { return goodsDao.findById(id); }
控制层写具体实现
相关阅读 >>
更多相关阅读请进入《sql》频道 >>
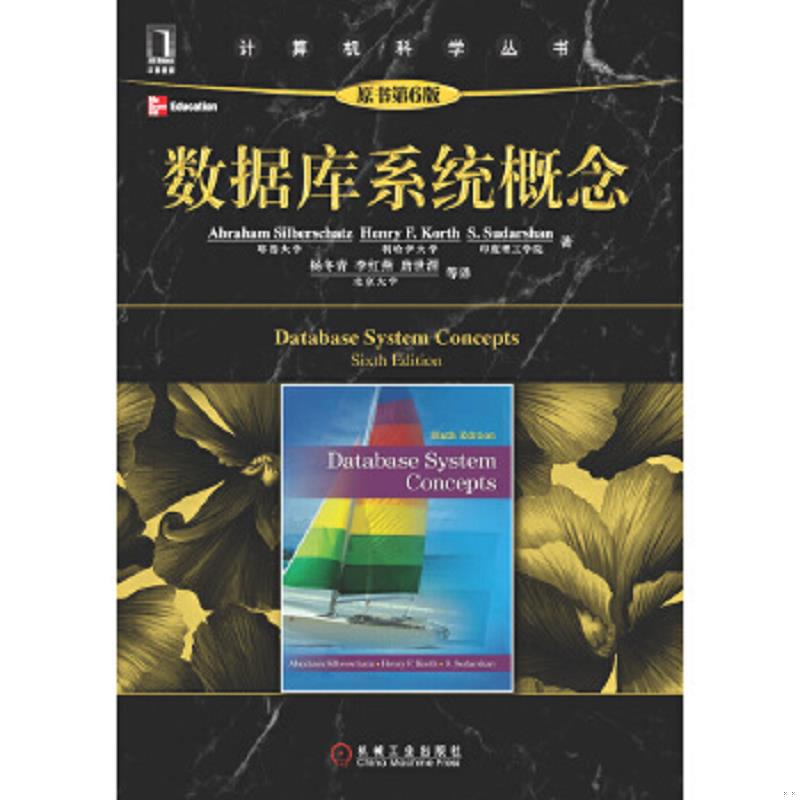
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。