本文整理自网络,侵删。
Android中基于Sqlite实现注册和登录功能,供大家参考,具体内容如下
前言
写这篇博客主要是为了巩固一下学的Sqlite知识以及梳理一下这个项目的逻辑
实现逻辑
项目的图片结构图如下
代码
user class
public class User { ? ? private String name; ? ?//用户名 ? ? private String password; ? ??//密码 ? ? public User(String name, String password) { ? ? ? ? this.name = name; ? ? ? ? this.password = password; ? ? } ? ? public String getName() { ? ? ? ? return name; ? ? } ? ? public void setName(String name) { ? ? ? ? this.name = name; ? ? } ? ? public String getPassword() { ? ? ? ? return password; ? ? } ? ? public void setPassword(String password) { ? ? ? ? this.password = password; ? ? } }
DBOpenHelper class
import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import java.util.ArrayList; public class DBOpenHelper extends SQLiteOpenHelper { ? ? private SQLiteDatabase db; ? ? public DBOpenHelper(Context context){//打开数据库 ? ? ? ? super(context,"db_test",null,1);//1:上下文,2:数据库名,3:允许我们查询数据时返回一个Cursor,4:当前数据库的版本号 ? ? ? ? db = getReadableDatabase(); ? ? } ? ? @Override ? ? public void onCreate(SQLiteDatabase db){//建表(user)语句 ? ? ? ? db.execSQL("CREATE TABLE IF NOT EXISTS user(" +//PRIMARY key 将id设为主键 ,AUTOINCREMENT 设置id列自为增长 ? ? ? ? ? ? ? ? "_id INTEGER PRIMARY KEY AUTOINCREMENT," + ? ? ? ? ? ? ? ? "name TEXT," + ? ? ? ? ? ? ? ? ? ? ? //text 文本类型 ? ? ? ? ? ? ? ? "password TEXT)"); ? ? } ? ? @Override ? ? public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion){//重写升级 ? ? ? ? db.execSQL("DROP TABLE IF EXISTS user"); ? ? ? ? onCreate(db); ? ? } ? ? public void add(String name,String password){//重写添加 ? ? ? ? db.execSQL("INSERT INTO user (name,password) VALUES(?,?)",new Object[]{name,password}); ? ? } ? ? public void delete(String name,String password){//重写删除 ? ? ? ? db.execSQL("DELETE FROM user WHERE name = AND password ="+name+password); ? ? } ? ? public void updata(String password){//重写更新 ? ? ? ? db.execSQL("UPDATE user SET password = ?",new Object[]{password}); ? ? } ? ? public ArrayList<User> getAllData(){//将表内信息返回成一个list ? ? ? ? ArrayList<User> list = new ArrayList<User>(); ? ? ? ? Cursor cursor = db.query("user",null,null,null,null,null,"name DESC");//1表名,2列,3行,4行,5指定列进行过滤,6进一步过滤。7得到的信息进行排序(desc逆序) ? ? ? ? while(cursor.moveToNext()){//一行一行遍历 ? ? ? ? ? ? String name = cursor.getString(cursor.getColumnIndex("name"));//移动到name列,读取出来 ? ? ? ? ? ? String password = cursor.getString(cursor.getColumnIndex("password")); ? ? ? ? ? ? list.add(new User(name,password));//添加到user 的list中 ? ? ? ? } ? ? ? ? return list;//把list返回 ? ? } }
activity_login.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" ? ? xmlns:app="http://schemas.android.com/apk/res-auto" ? ? xmlns:tools="http://schemas.android.com/tools" ? ? android:layout_width="match_parent" ? ? android:layout_height="match_parent" ? ? android:background="#eeeeee" ? ? tools:context=".LoginActivity"> ? ? <RelativeLayout ? ? ? ? android:id="@+id/rl_loginactivity_top" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="70dp" ? > ? ? ? ? <ImageView ? ? ? ? ? ? android:id="@+id/iv_loginactivity_back" ? ? ? ? ? ? android:layout_width="30dp" ? ? ? ? ? ? android:layout_height="30dp" ? ? ? ? ? ? android:layout_alignParentTop="true" ? ? ? ? ? ? android:layout_marginLeft="10dp" ? ? ? ? ? ? android:layout_marginTop="20dp" ? ? ? ? ? ? android:clickable="true" /> ? ? ? ? <TextView ? ? ? ? ? ? android:id="@+id/tv_loginactivity_login" ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:text="登录" ? ? ? ? ? ? android:textColor="#3A5FCD" ? ? ? ? ? ? android:textSize="20dp" ? ? ? ? ? ? android:layout_toRightOf="@+id/iv_loginactivity_back" ? ? ? ? ? ? android:layout_centerVertical="true" ? ? ? ? ? ? android:layout_alignParentLeft="true" ? ? ? ? ? ? android:layout_marginLeft="30dp" ? ? ? ? ? ? /> ? ? ? ? <TextView ? ? ? ? ? ? android:id="@+id/tv_loginactivity_register" ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:text="注册" ? ? ? ? ? ? android:textColor="#3A5FCD" ? ? ? ? ? ? android:textSize="20dp" ? ? ? ? ? ? android:layout_centerVertical="true" ? ? ? ? ? ? android:layout_alignParentRight="true" ? ? ? ? ? ? android:layout_marginRight="30dp" ? ? ? ? ? ? android:clickable="true" ? ? ? ? ? ? android:onClick="onClick" ? ? ? ? ? ? /> ? ? </RelativeLayout> ? ? <LinearLayout ? ? ? ? android:id="@+id/ll_loginactivity_two" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:orientation="vertical" ? ? ? ? android:layout_below="@+id/rl_loginactivity_top" ? ? ? ? android:layout_marginTop="10dp" ? ? ? ? android:layout_marginLeft="5dp" ? ? ? ? android:layout_marginRight="5dp" ? ? ? ? > ? ? ? ? <LinearLayout ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:orientation="horizontal"> ? ? ? ? ? ? <TextView ? ? ? ? ? ? ? ? android:id="@+id/tv_loginactivity_username" ? ? ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:text="用户名:"/> ? ? ? ? ? ? <EditText ? ? ? ? ? ? ? ? android:id="@+id/et_loginactivity_username" ? ? ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:hint="手机号/邮箱/用户名"/> ? ? ? ? </LinearLayout> ? ? ? ? <LinearLayout ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:orientation="horizontal"> ? ? ? ? ? ? <TextView ? ? ? ? ? ? ? ? android:id="@+id/tv_loginactivity_password" ? ? ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:text="密 ? ?码:"/> ? ? ? ? ? ? <EditText ? ? ? ? ? ? ? ? android:id="@+id/et_loginactivity_password" ? ? ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:hint="登录密码" ? ? ? ? ? ? ? ? android:inputType="textPassword"/> ? ? ? ? </LinearLayout> ? ? </LinearLayout> ? ? <Button ? ? ? ? android:id="@+id/bt_loginactivity_login" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:layout_below="@+id/ll_loginactivity_two" ? ? ? ? android:layout_marginTop="10dp" ? ? ? ? android:layout_marginLeft="5dp" ? ? ? ? android:layout_marginRight="5dp" ? ? ? ? android:text="登录" ? ? ? ? android:textColor="#3A5FCD" ? ? ? ? android:gravity="center" ? ? ? ? android:onClick="onClick" ? ? ? ? /> </RelativeLayout>
LoginActivity
import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.content.Intent; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import java.util.ArrayList; public class LoginActivity extends AppCompatActivity implements View.OnClickListener { ? ? private DBOpenHelper mDBOpenHelper; ? ? private EditText mEtLoginactivityUsername; ? ? private EditText mEtLoginactivityPassword; ? ? @Override ? ? protected void onCreate(Bundle savedInstanceState) { ? ? ? ? super.onCreate(savedInstanceState); ? ? ? ? setContentView(R.layout.activity_login); ? ? ? ? initView(); ? ? ? ? mDBOpenHelper = new DBOpenHelper(this); ? ? } ? ? private void initView() { ? ? ? ? // 初始化控件 ? ? ? ? mEtLoginactivityUsername = findViewById(R.id.et_loginactivity_username); ? ? ? ? mEtLoginactivityPassword = findViewById(R.id.et_loginactivity_password); ? ? ? ? // 设置点击事件监听器 ? ? } ? ? public void onClick(View view) { ? ? ? ? switch (view.getId()) { ? ? ? ? ? ? // 跳转到注册界面 ? ? ? ? ? ? case R.id.tv_loginactivity_register: ? ? ? ? ? ? ? ? startActivity(new Intent(this, RegisterActivity.class)); ? ? ? ? ? ? ? ? finish(); ? ? ? ? ? ? ? ? break; ? ? ? ? ? ? case R.id.bt_loginactivity_login: ? ? ? ? ? ? ? ? String name = mEtLoginactivityUsername.getText().toString().trim();//.trim()删除两边的空格 ? ? ? ? ? ? ? ? String password = mEtLoginactivityPassword.getText().toString().trim(); ? ? ? ? ? ? ? ? if (!TextUtils.isEmpty(name) && !TextUtils.isEmpty(password)) {//TextUtils.isEmpty()输入框是空值或者你就敲了几下空格键该方法都会返回true ? ? ? ? ? ? ? ? ? ? ArrayList<User> data = mDBOpenHelper.getAllData();//data为获取的user表内的user信息 ? ? ? ? ? ? ? ? ? ? boolean match = false; ? ? ? ? ? ? ? ? ? ? for (int i = 0; i < data.size(); i++) {//遍历比较 ? ? ? ? ? ? ? ? ? ? ? ? User user = data.get(i);//获取data里的第i个user信息 ? ? ? ? ? ? ? ? ? ? ? ? if (name.equals(user.getName()) && password.equals(user.getPassword())) {//将信息与输入的信息进行对比 ? ? ? ? ? ? ? ? ? ? ? ? ? ? match = true; ? ? ? ? ? ? ? ? ? ? ? ? ? ? break; ? ? ? ? ? ? ? ? ? ? ? ? } else { ? ? ? ? ? ? ? ? ? ? ? ? ? ? match = false; ? ? ? ? ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? ? ? if (match) { ? ? ? ? ? ? ? ? ? ? ? ? Toast.makeText(this, "登录成功", Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? ? ? ? ? Intent intent = new Intent(this, MainActivity.class); ? ? ? ? ? ? ? ? ? ? ? ? startActivity(intent); ? ? ? ? ? ? ? ? ? ? ? ? finish();//销毁此Activity ? ? ? ? ? ? ? ? ? ? } else { ? ? ? ? ? ? ? ? ? ? ? ? Toast.makeText(this, "用户名或密码不正确,请重新输入", Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? } else { ? ? ? ? ? ? ? ? ? ? Toast.makeText(this, "请输入你的用户名或密码", Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? break; ? ? ? ? } ? ? } }
activity_register.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" ? ? xmlns:app="http://schemas.android.com/apk/res-auto" ? ? xmlns:tools="http://schemas.android.com/tools" ? ? android:layout_width="match_parent" ? ? android:layout_height="match_parent" ? ? android:background="#eeeeee" ? ? tools:context=".RegisterActivity"> ? ? <RelativeLayout ? ? ? ? android:id="@+id/rl_registeractivity_top" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="70dp" ? ? ? ? > ? ? ? ? <ImageView ? ? ? ? ? ? android:id="@+id/iv_registeractivity_back" ? ? ? ? ? ? android:layout_width="30dp" ? ? ? ? ? ? android:layout_height="30dp" ? ? ? ? ? ? android:layout_centerVertical="true" ? ? ? ? ? ? android:layout_marginLeft="10dp" ? ? ? ? ? ? android:clickable="true" ? ? ? ? ? ? /> ? ? ? ? <TextView ? ? ? ? ? ? android:id="@+id/tv_registeractivity_register" ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:text="注册" ? ? ? ? ? ? android:textColor="#3A5FCD" ? ? ? ? ? ? android:textSize="20dp" ? ? ? ? ? ? android:layout_centerVertical="true" ? ? ? ? ? ? android:layout_marginLeft="20dp" ? ? ? ? ? ? /> ? ? </RelativeLayout> ? ? <LinearLayout ? ? ? ? android:id="@+id/ll_registeractivity_body" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:orientation="vertical" ? ? ? ? android:layout_below="@+id/rl_registeractivity_top" ? ? ? ? android:layout_marginTop="10dp" ? ? ? ? android:layout_marginLeft="5dp" ? ? ? ? android:layout_marginRight="5dp" ? ? ? ? > ? ? ? ? <!-- 第一个文本编辑框 ?输入用户名 --> ? ? ? ? <LinearLayout ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:orientation="horizontal"> ? ? ? ? ? ? <TextView ? ? ? ? ? ? ? ? android:id="@+id/tv_registeractivity_username" ? ? ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:text="用户名:"/> ? ? ? ? ? ? <EditText ? ? ? ? ? ? ? ? android:id="@+id/et_registeractivity_username" ? ? ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? ? ? android:layout_height="50dp" ? ? ? ? ? ? ? ? android:hint="请输入用户名" ? ? ? ? ? ? ? ? android:gravity="center_vertical" ? ? ? ? ? ? ? ? android:layout_marginLeft="10dp" ? ? ? ? ? ? ? ? /> ? ? ? ? </LinearLayout> ? ? ? ? <!-- 第二个文本编辑框 ?输入密码 --> ? ? ? ? <LinearLayout ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:orientation="horizontal"> ? ? ? ? ? ? <TextView ? ? ? ? ? ? ? ? android:id="@+id/tv_registeractivity_password1" ? ? ? ? ? ? ? ? android:layout_width="wrap_content" ? ? ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? ? ? android:text="密 ? ?码:"/> ? ? ? ? ? ? <EditText ? ? ? ? ? ? ? ? android:id="@+id/et_registeractivity_password" ? ? ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? ? ? android:layout_height="50dp" ? ? ? ? ? ? ? ? android:gravity="center_vertical" ? ? ? ? ? ? ? ? android:layout_marginLeft="10dp" ? ? ? ? ? ? ? ? android:inputType="textPassword" ? ? ? ? ? ? ? ? android:hint="请输入密码" /> ? ? ? ? </LinearLayout> ? ? ? ? <LinearLayout ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="wrap_content" ? ? ? ? ? ? android:layout_marginRight="15dp" ? ? ? ? ? ? android:layout_marginTop="10dp" ? ? ? ? ? ? android:orientation="horizontal" /> ? ? ? ? <!-- 注册按钮 --> ? ? ? ? <Button ? ? ? ? ? ? android:id="@+id/bt_registeractivity_register" ? ? ? ? ? ? android:layout_width="match_parent" ? ? ? ? ? ? android:layout_height="50dp" ? ? ? ? ? ? android:layout_marginLeft="5dp" ? ? ? ? ? ? android:textColor="#3A5FCD" ? ? ? ? ? ? android:text="注册" ? ? ? ? ? ? android:layout_marginTop="40dp" ? ? ? ? ? ? android:onClick="onClick" ? ? ? ? ? ? /> ? ? </LinearLayout> </RelativeLayout>
RegisterActivity
import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.ImageView; import android.widget.LinearLayout; import android.widget.RelativeLayout; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; public class RegisterActivity extends AppCompatActivity implements View.OnClickListener { ? ? private DBOpenHelper mDBOpenHelper; ? ? private Button mBtRegisteractivityRegister; ? ? private ImageView mIvRegisteractivityBack; ? ? private EditText mEtRegisteractivityUsername; ? ? private EditText mEtRegisteractivityPassword; ? ? @Override ? ? protected void onCreate(Bundle savedInstanceState) { ? ? ? ? super.onCreate(savedInstanceState); ? ? ? ? setContentView(R.layout.activity_register); ? ? ? ? initView(); ? ? ? ? mDBOpenHelper = new DBOpenHelper(this); ? ? } ? ? private void initView(){ ? ? ? ? mEtRegisteractivityUsername = findViewById(R.id.et_registeractivity_username); ? ? ? ? mEtRegisteractivityPassword = findViewById(R.id.et_registeractivity_password); } ? ? public void onClick(View view) { ? ? ? ? switch (view.getId()) { ? ? ? ? ? ? case R.id.iv_registeractivity_back: //返回登录页面 ? ? ? ? ? ? ? ? Intent intent1 = new Intent(this, LoginActivity.class); ? ? ? ? ? ? ? ? startActivity(intent1); ? ? ? ? ? ? ? ? finish(); ? ? ? ? ? ? ? ? break; ? ? ? ? ? ? case R.id.bt_registeractivity_register: ? ?//注册按钮 ? ? ? ? ? ? ? ? //获取用户输入的用户名、密码、验证码 ? ? ? ? ? ? ? ? String username = mEtRegisteractivityUsername.getText().toString().trim(); ? ? ? ? ? ? ? ? String password = mEtRegisteractivityPassword.getText().toString().trim(); ? ? ? ? ? ? ? ? //注册验证 ? ? ? ? ? ? ? ? if (!TextUtils.isEmpty(username) && !TextUtils.isEmpty(password)) { ? ? ? ? ? ? ? ? ? ? mDBOpenHelper.add(username, password);//将用户名和密码加入到数据库的表内中 ? ? ? ? ? ? ? ? ? ? Intent intent2 = new Intent(this, LoginActivity.class); ? ? ? ? ? ? ? ? ? ? startActivity(intent2); ? ? ? ? ? ? ? ? ? ? finish(); ? ? ? ? ? ? ? ? ? ? Toast.makeText(this, "验证通过,注册成功", Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? } else { ? ? ? ? ? ? ? ? ? ? Toast.makeText(this, "未完善信息,注册失败", Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? break; ? ? ? ? } ? ? } }
实现效果
标签:SQLite
相关阅读 >>
android studio 使用adb 命令传递文件到android 设备的方法
android studio如何获取Sqlite数据并显示到listview上
python Sqlite3 判断cursor的结果是否为空的案例
cc++qt数据库sqlrelationaltable关联表详解
更多相关阅读请进入《Sqlite》频道 >>
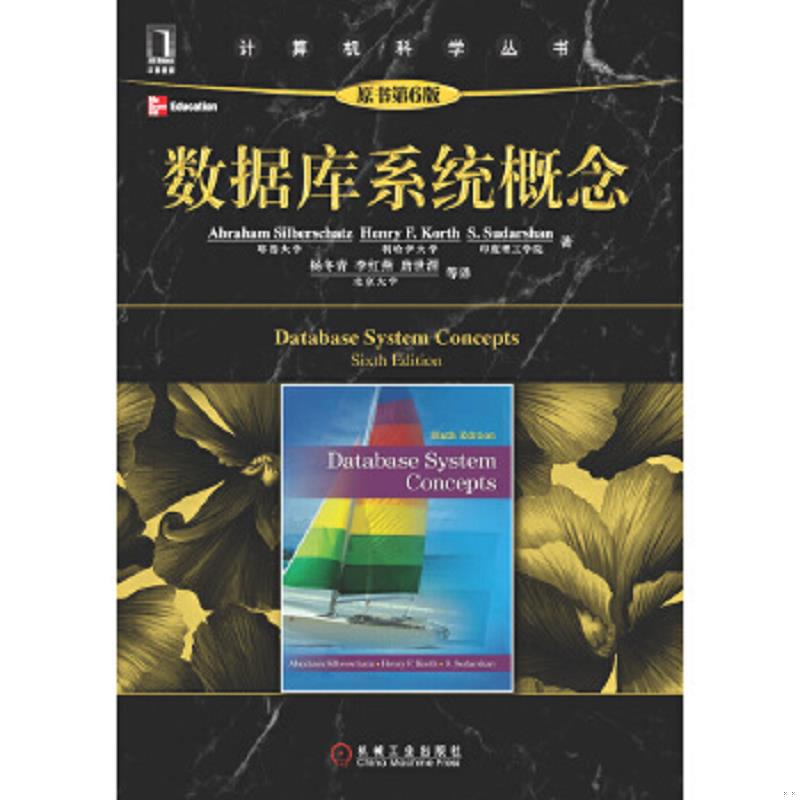
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。