本文整理自网络,侵删。
本文实例为大家分享了AndriodStudio利用ListView和数据库实现简单学生管理的具体代码,供大家参考,具体内容如下
数据库的创建
package com.example.myapp; ? import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.widget.Toast; ? public class DbHelper extends SQLiteOpenHelper { ? ? final String create_table="CREATE TABLE Student (_id integer primary key autoincrement,xm text,xh text,bj text,zy text,cj text)"; ? ? final String create_register="CREATE TABLE Register (_id integer primary key autoincrement,xm text,xh text)"; //创建两张表,一张student,一张register表 ? ? Context context; ? ? public DbHelper(Context context,String dbname,int version){ ? ? ? ? super(context,dbname,null,version); ? ? ? ? this.context=context;//上下文 ? ? } ? ? @Override ? ? public void onCreate(SQLiteDatabase db) {db.execSQL(create_table); db.execSQL(create_register);} ? ? ? @Override ? ? public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { ? ? ? ? db.execSQL("drop table if exists Student"); ? ? ? ? db.execSQL("drop table if exists Register"); ? ? ? ? db.execSQL(create_table); ? ? ? ? db.execSQL(create_register); //完成创建 ? ? } // 对表进行数据的插入方法 ? ? public void insert(String tableName, ContentValues values){ ? ? ? ? SQLiteDatabase db = getReadableDatabase(); ? ? ? ? db.insert(tableName,null,values); ? ? ? ? Toast.makeText(context,"成功插入数据!",Toast.LENGTH_SHORT).show(); ? ? } //查询表中所有 ? ? public Cursor queryAll(String tableName){ ? ? ? ? SQLiteDatabase db = getReadableDatabase(); ? ? ? ? Cursor cursor = db.query(tableName,null,null,null,null,null,null); ? ? ? ? return cursor; ? ? } //对表中的姓名和学号进行单独的查询 ? ? public Boolean queryByStudentXhAndXm(String tableName,String xm,String xh){ ? ? ? ? SQLiteDatabase db = getReadableDatabase(); ? ? ? ? Cursor cursor = db.query(tableName,new String[]{"xm,xh"},"xm=? and xh=?",new String[]{xm,xh},null,null,null); ? ? ? ? if (cursor.moveToFirst()) { ? ? ? ? ? ? return true; ? ? ? ? }else { ? ? ? ? ? ? return false; ? ? ? ? } ? ? } //删除表中数据的方法 ? ? public void delStudent(String id){ ? ? ? ? SQLiteDatabase db = getWritableDatabase(); ? ? ? ? db.delete("Student","_id=?",new String[]{id}); ? ? } //对表进行更新 ? ? public void ?updateStudent(String id,ContentValues values){ ? ? ? ? SQLiteDatabase db = getWritableDatabase(); ? ? ? ? db.update("Student",values,"_id=?",new String[]{id}); ? ? } }
创建了两张表格,一张用来储存学生信息,Student表:id(主键),姓名,学号,班级,专业,成绩。
一张register表:id(主键),姓名,学号。用来储存登录信息
其余类和其布局文件
MainActivity.class(登录界面)
package com.example.myapp; ? import androidx.appcompat.app.AppCompatActivity; ? import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; ? public class MainActivity extends AppCompatActivity { ? ? EditText et1,et2; ? ? Button btn1,btn2; ? ? DbHelper dbHelper; ? ? @Override ? ? protected void onCreate(Bundle savedInstanceState) { ? ? ? ? super.onCreate(savedInstanceState); ? ? ? ? dbHelper=new DbHelper(MainActivity.this,"MyDataBase,",3); ? ? ? ? setContentView(R.layout.activity_main); ? ? ? ? et1=findViewById(R.id.et1); ? ? ? ? et2=findViewById(R.id.et2); ? ? ? ? btn1=findViewById(R.id.dl); ? ? ? ? btn2=findViewById(R.id.zc); ? ? ? ? ? btn1.setOnClickListener(new View.OnClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onClick(View v) { ? ? ? ? ? ? ? ? String xm=et1.getText().toString(); ? ? ? ? ? ? ? ? String xh=et2.getText().toString(); ? ? ? ? ? ? ? ? if (xm.isEmpty()||xh.isEmpty()){ ? ? ? ? ? ? ? ? ? ? Toast.makeText(MainActivity.this,"姓名或者学号不可为空",Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? if (dbHelper.queryByStudentXhAndXm("Register",xm,xh)){ ? ? ? ? ? ? ? ? ? ? Intent intent=new Intent(MainActivity.this,Manage.class); ? ? ? ? ? ? ? ? ? ? startActivity(intent); ? ? ? ? ? ? ? ? } ? ? ? ? ? ? } ? ? ? ? }); ? ? ? ? btn2.setOnClickListener(new View.OnClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onClick(View v) { ? ? ? ? ? ? ? ? Intent intent=new Intent(MainActivity.this,Register.class); ? ? ? ? ? ? ? ? startActivity(intent); ? ? ? ? ? ? } ? ? ? ? }); ? ? } }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" ? ? xmlns:app="http://schemas.android.com/apk/res-auto" ? ? xmlns:tools="http://schemas.android.com/tools" ? ? android:layout_width="match_parent" ? ? android:layout_height="match_parent" ? ? android:orientation="vertical" ? ? tools:context=".MainActivity"> ? ? ? <EditText ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:id="@+id/et1" ? ? ? ? android:hint="输入姓名"/> ? ? <EditText ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:id="@+id/et2" ? ? ? ? android:hint="学号"/> ? ? <Button ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:id="@+id/dl" ? ? ? ? android:text="登录"/> ? ? <Button ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:text="注册" ? ? ? ? android:id="@+id/zc"/> ? </LinearLayout>
Register.class(注册界面)
package com.example.myapp; ? import androidx.appcompat.app.AppCompatActivity; ? import android.content.ContentValues; import android.content.Intent; import android.database.Cursor; import android.os.Bundle; import android.text.TextUtils; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; ? public class Register extends AppCompatActivity { ? ? EditText et1,et2; ? ? Button btn1,btn2; ? ? DbHelper dbHelper; ? ? TextView show; ? ? ? @Override ? ? protected void onCreate(Bundle savedInstanceState) { ? ? ? ? super.onCreate(savedInstanceState); ? ? ? ? setContentView(R.layout.activity_register); ? ? ? ? dbHelper=new DbHelper(Register.this,"MyDataBase,",3); ? ? ? ? et1=findViewById(R.id.xm_zc); ? ? ? ? et2=findViewById(R.id.xh_zc); ? ? ? ? show=findViewById(R.id.tv_show); ? ? ? ? btn1=findViewById(R.id.qd_zc); ? ? ? ? btn2=findViewById(R.id.fh); ? ? ? ? ? btn1.setOnClickListener(new View.OnClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onClick(View v) { ? ? ? ? ? ? ? ? String xm=et1.getText().toString(); ? ? ? ? ? ? ? ? String xh=et2.getText().toString(); ? ? ? ? ? ? ? ? if(TextUtils.isEmpty(xm)||TextUtils.isEmpty(xh)){ ? ? ? ? ? ? ? ? ? ? Toast.makeText(Register.this,"姓名或者学号不能为空",Toast.LENGTH_SHORT).show(); ? ? ? ? ? ? ? ? ? ? return; ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? ContentValues values = new ContentValues(); ? ? ? ? ? ? ? ? values.put("xm",xm); ? ? ? ? ? ? ? ? values.put("xh",xh); ? ? ? ? ? ? ? ? dbHelper.insert("Register",values); ? ? ? ? ? ? ? ? showUser(); ? ? ? ? ? ? } ? ? ? ? }); ? ? ? ? btn2.setOnClickListener(new View.OnClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onClick(View v) { ? ? ? ? ? ? ? ? Intent intent=new Intent(Register.this,MainActivity.class); ? ? ? ? ? ? ? ? startActivity(intent); ? ? ? ? ? ? } ? ? ? ? }); ? ? } ? ? public void showUser(){ ? ? ? ? Cursor cursor = dbHelper.queryAll("Register"); ? ? ? ? String str = "_id ? ? ? ?xm ? ? ? ? xh\n"; ? ? ? ? if (cursor.moveToFirst()) ? ? ? ? ? ? while (cursor.moveToNext()){ ? ? ? ? ? ? ? ? str += cursor.getString(0)+" ? ? ?"; ? ? ? ? ? ? ? ? str += cursor.getString(1)+" ? ? ?"; ? ? ? ? ? ? ? ? str += cursor.getString(2)+"\n"; ? ? ? ? ? ? }; ? ? ? ? show.setText(str); ? ? } }
activity_register.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" ? ? xmlns:app="http://schemas.android.com/apk/res-auto" ? ? xmlns:tools="http://schemas.android.com/tools" ? ? android:layout_width="match_parent" ? ? android:layout_height="match_parent" ? ? android:orientation="vertical" ? ? tools:context=".Register"> ? ? <EditText ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:id="@+id/xm_zc" ? ? ? ? android:hint="输入姓名"/> ? ? <EditText ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:id="@+id/xh_zc" ? ? ? ? android:hint="输入学号"/> ? ? <Button ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:text="确认注册" ? ? ? ? android:id="@+id/qd_zc"/> ? ? <Button ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:text="返回上一层" ? ? ? ? android:id="@+id/fh"/> ? ? <TextView ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="match_parent" ? ? ? ? android:id="@+id/tv_show"/> ? </LinearLayout>
Manage.class(管理界面)
package com.example.myapp; ? import androidx.appcompat.app.AlertDialog; import androidx.appcompat.app.AppCompatActivity; ? import android.content.ContentValues; import android.content.DialogInterface; import android.content.Intent; import android.database.Cursor; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.Button; import android.widget.ListView; import android.widget.SimpleCursorAdapter; ? public class Manage extends AppCompatActivity { ? ? ListView listView; ? ? Button btn; ? ? DbHelper dbHelper; ? ? ? @Override ? ? protected void onCreate(Bundle savedInstanceState) { ? ? ? ? super.onCreate(savedInstanceState); ? ? ? ? setContentView(R.layout.activity_manage); ? ? ? ? AlertDialog.Builder builder = new AlertDialog.Builder(Manage.this); ? ? ? ? dbHelper = new DbHelper(Manage.this,"MyDataBase,",3); ? ? ? ? listView=findViewById(R.id.list); ? ? ? ? dbHelper.getWritableDatabase(); ? ? ? ? renderListView(); ? ? ? ? btn=findViewById(R.id.new_list); ? ? ? ? btn.setOnClickListener(new View.OnClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onClick(View v) { ? ? ? ? ? ? ? ? Intent intent=new Intent(Manage.this,NewStudent.class); ? ? ? ? ? ? ? ? startActivityForResult(intent,1); ? ? ? ? ? ? } ? ? ? ? }); ? ? ? ? listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public void onItemClick(AdapterView<?> parent, View view, int position, long idi) { ? ? ? ? ? ? ? ? Cursor cursor = dbHelper.queryAll("Student"); ? ? ? ? ? ? ? ? cursor.move(position+1); ? ? ? ? ? ? ? ? String id = cursor.getString(cursor.getColumnIndex("_id")); ? ? ? ? ? ? ? ? String xm = cursor.getString(cursor.getColumnIndex("xm")); ? ? ? ? ? ? ? ? String xh = cursor.getString(cursor.getColumnIndex("xh")); ? ? ? ? ? ? ? ? String bj = cursor.getString(cursor.getColumnIndex("bj")); ? ? ? ? ? ? ? ? String zy = cursor.getString(cursor.getColumnIndex("zy")); ? ? ? ? ? ? ? ? String cj = cursor.getString(cursor.getColumnIndex("cj")); ? ? ? ? ? ? ? ? Intent intent = new Intent(Manage.this,NewStudent.class); ? ? ? ? ? ? ? ? intent.putExtra("id",id); ? ? ? ? ? ? ? ? intent.putExtra("xm",xm); ? ? ? ? ? ? ? ? intent.putExtra("xh",xh); ? ? ? ? ? ? ? ? intent.putExtra("bj",bj); ? ? ? ? ? ? ? ? intent.putExtra("zy",zy); ? ? ? ? ? ? ? ? intent.putExtra("cj",cj); ? ? ? ? ? ? ? ? startActivityForResult(intent,2); ? ? ? ? ? ? } ? ? ? ? }); ? ? ? ? listView.setOnItemLongClickListener(new AdapterView.OnItemLongClickListener() { ? ? ? ? ? ? @Override ? ? ? ? ? ? public boolean onItemLongClick(AdapterView<?> parent, View view, int position, long idi) { ? ? ? ? ? ? ? ? Cursor cursor = dbHelper.queryAll("Student"); ? ? ? ? ? ? ? ? cursor.move(position+1); ? ? ? ? ? ? ? ? String id = cursor.getString(cursor.getColumnIndex("_id"));//getColumnIndex("_id")得到这一列 ? ? ? ? ? ? ? ? String xm = cursor.getString(cursor.getColumnIndex("xm")); ? ? ? ? ? ? ? ? builder.setTitle("删除确认").setMessage("是否确认删除学生"+xm); ? ? ? ? ? ? ? ? builder.setPositiveButton("确定", new DialogInterface.OnClickListener() { ? ? ? ? ? ? ? ? ? ? @Override ? ? ? ? ? ? ? ? ? ? public void onClick(DialogInterface dialog, int which) { ? ? ? ? ? ? ? ? ? ? ? ? dbHelper.delStudent(id); ? ? ? ? ? ? ? ? ? ? ? ? renderListView(); ? ? ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? }); ? ? ? ? ? ? ? ? builder.show(); ? ? ? ? ? ? ? ? return true; ? ? ? ? ? ? } ? ? ? ? }); ? ? } ? ? private void renderListView() { ? ? ? ? Cursor cursor = dbHelper.queryAll("Student"); ? ? ? ? String from[] = new String[]{"_id", "xm", "xh","bj","zy","cj"}; ? ? ? ? int to[] = new int[]{R.id.id,R.id.xm, R.id.xh, R.id.bj, R.id.zy, R.id.cj}; ? ? ? ? SimpleCursorAdapter adapter = new SimpleCursorAdapter(this, R.layout.listview, cursor, from, to, 0); ? ? ? ? ListView listView = findViewById(R.id.list); ? ? ? ? listView.setAdapter(adapter); ? ? } ? ? @Override ? ? protected ?void onActivityResult(int reqCode,int resultCode,Intent intent){ ? ? ? ? super.onActivityResult(reqCode,resultCode,intent); ? ? ? ? if (resultCode==RESULT_OK){ ? ? ? ? ? ? String xm = intent.getStringExtra("xm"); ? ? ? ? ? ? String xh = intent.getStringExtra("xh"); ? ? ? ? ? ? String bj = intent.getStringExtra("bj"); ? ? ? ? ? ? String zy = intent.getStringExtra("zy"); ? ? ? ? ? ? String cj = intent.getStringExtra("cj"); ? ? ? ? ? ? dbHelper.getWritableDatabase(); ? ? ? ? ? ? ContentValues values = new ContentValues(); ? ? ? ? ? ? values.put("xm",xm); ? ? ? ? ? ? values.put("xh",xh); ? ? ? ? ? ? values.put("bj",bj); ? ? ? ? ? ? values.put("zy",zy); ? ? ? ? ? ? values.put("cj",cj); ? ? ? ? ? ? if (reqCode==1) ? ? ? ? ? ? ? ? dbHelper.insert("Student",values); ? ? ? ? ? ? else if (reqCode==2){ ? ? ? ? ? ? ? ? String id = intent.getStringExtra("id"); ? ? ? ? ? ? ? ? dbHelper.updateStudent(id,values); ? ? ? ? ? ? } ? ? ? ? ? ? renderListView(); ? ? ? ? } ? ? } }
activity_manage.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" ? ? xmlns:app="http://schemas.android.com/apk/res-auto" ? ? xmlns:tools="http://schemas.android.com/tools" ? ? android:layout_width="match_parent" ? ? android:layout_height="match_parent" ? ? android:orientation="vertical" ? ? tools:context=".Manage"> ? ? ? <ListView ? ? ? ? android:id="@+id/list" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" /> ? ? ? <Button ? ? ? ? android:id="@+id/new_list" ? ? ? ? android:layout_width="match_parent" ? ? ? ? android:layout_height="wrap_content" ? ? ? ? android:text="添加新学生" /> ? </LinearLayout>
对应的listview的布局文件,listview.xml
相关阅读 >>
python web框架之django框架model基础详解
开源 5 款超好用的数据库 gui 带你玩转 mongodb、redis、sql 数据库(推荐)
更多相关阅读请进入《Sqlite》频道 >>
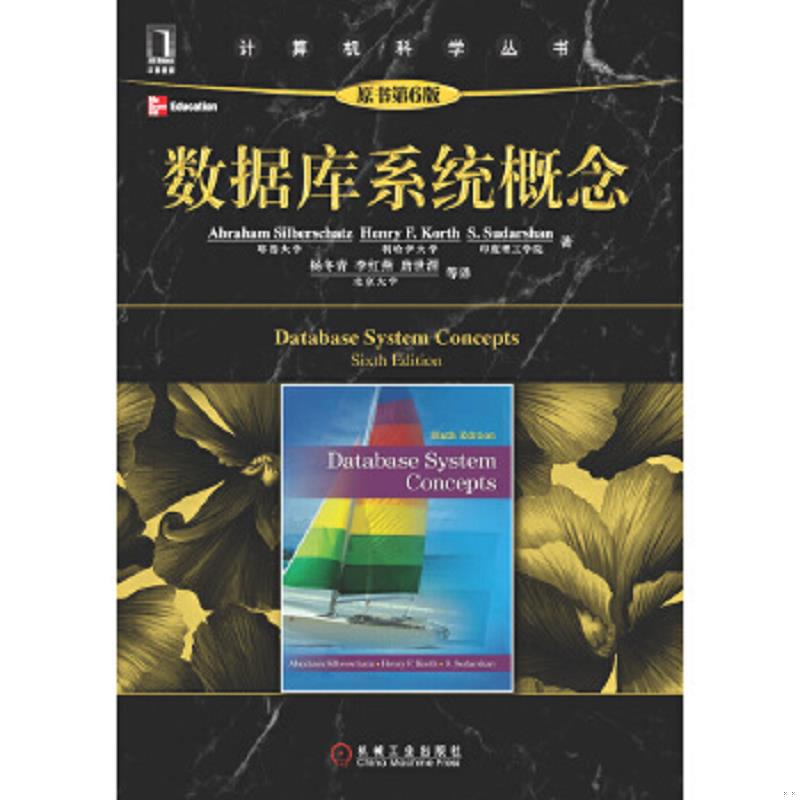
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。