本文摘自php中文网,作者爱喝马黛茶的安东尼,侵删。
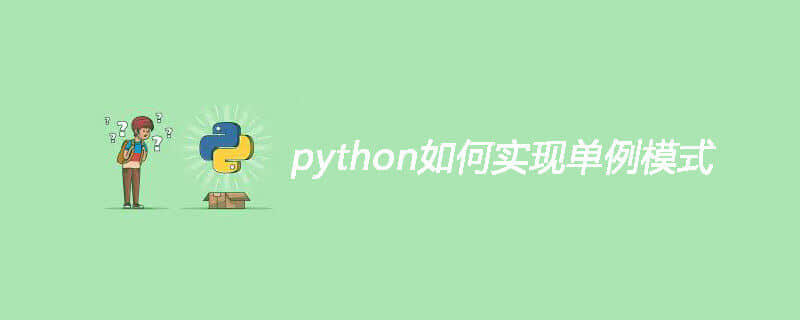
python如何实现单例模式?下面给大家带来七种不同的方法:
一:staticmethod
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 | class Singleton(object):
instance = None
def __init__(self):
raise SyntaxError( 'can not instance, please use get_instance' )
def get_instance():
if Singleton.instance is None:
Singleton.instance = object.__new__(Singleton)
return Singleton.instance
a = Singleton.get_instance()
b = Singleton.get_instance()
print ( 'a id=' , id(a))
print ( 'b id=' , id(b))
|
该方法的要点是在__init__抛出异常,禁止通过类来实例化,只能通过静态get_instance函数来获取实例;因为不能通过类来实例化,所以静态get_instance函数中可以通过父类object.__new__来实例化。
二:classmethod
和方法一类似,代码:
1 2 3 4 5 6 7 8 9 10 11 12 | class Singleton(object):
instance = None
def __init__(self):
raise SyntaxError( 'can not instance, please use get_instance' )
def get_instance(cls):
if Singleton.instance is None:
Singleton.instance = object.__new__(Singleton)
return Singleton.instance
a = Singleton.get_instance()
b = Singleton.get_instance()
print ( 'a id=' , id(a))
print ( 'b id=' , id(b))
|
该方法的要点是在__init__抛出异常,禁止通过类来实例化,只能通过静态get_instance函数来获取实例;因为不能通过类来实例化,所以静态get_instance函数中可以通过父类object.__new__来实例化。
三:类属性方法
和方法一类似, 代码:
1 2 3 4 5 6 7 8 9 10 11 12 | class Singleton(object):
instance = None
def __init__(self):
raise SyntaxError( 'can not instance, please use get_instance' )
def get_instance():
if Singleton.instance is None:
Singleton.instance = object.__new__(Singleton)
return Singleton.instance
a = Singleton.get_instance()
b = Singleton.get_instance()
print (id(a))
print (id(b))
|
该方法的要点是在__init__抛出异常,禁止通过类来实例化,只能通过静态get_instance函数来获取实例;因为不能通过类来实例化,所以静态get_instance函数中可以通过父类object.__new__来实例化。
四:__new__
常见的方法, 代码如下:
1 2 3 4 5 6 7 8 9 10 11 | class Singleton(object):
instance = None
def __new__(cls, *args, **kw):
if not cls.instance:
# cls.instance = object.__new__(cls, *args)
cls.instance = super(Singleton, cls).__new__(cls, *args, **kw)
return cls.instance
a = Singleton()
b = Singleton()
print (id(a))
print (id(b))
|
相关推荐:《Python视频教程》
五:装饰器
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | def Singleton(cls):
instances = {}
def getinstance():
if cls not in instances:
instances[cls] = cls()
return instances[cls]
return getinstance
class MyClass:
pass
a = MyClass()
b = MyClass()
c = MyClass()
print (id(a))
print (id(b))
print (id(c))
|
六:元类
python2版:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | class Singleton(type):
def __init__(cls, name, bases, dct):
super(Singleton, cls).__init__(name, bases, dct)
cls.instance = None
def __call__(cls, *args):
if cls.instance is None:
cls.instance = super(Singleton, cls).__call__(*args)
return cls.instance
class MyClass(object):
__metaclass__ = Singleton
a = MyClass()
b = MyClass()
c = MyClass()
print (id(a))
print (id(b))
print (id(c))
print (a is b)
print (a is c)
|
或者:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | class Singleton(type):
def __new__(cls, name, bases, attrs):
attrs[ "_instance" ] = None
return super(Singleton, cls).__new__(cls, name, bases, attrs)
def __call__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = super(Singleton, cls).__call__(*args, **kwargs)
return cls._instance
class Foo(object):
__metaclass__ = Singleton
x = Foo()
y = Foo()
print (id(x))
print (id(y))
|
python3版:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | class Singleton(type):
def __new__(cls, name, bases, attrs):
attrs[ 'instance' ] = None
return super(Singleton, cls).__new__(cls, name, bases, attrs)
def __call__(cls, *args, **kwargs):
if cls.instance is None:
cls.instance = super(Singleton, cls).__call__(*args, **kwargs)
return cls.instance
class Foo(metaclass=Singleton):
pass
x = Foo()
y = Foo()
print (id(x))
print (id(y))
|
七:名字覆盖
代码如下:
1 2 3 4 5 6 7 8 9 10 11 | class Singleton(object):
def foo(self):
print ( 'foo' )
def __call__(self):
return self
Singleton = Singleton()
Singleton.foo()
a = Singleton()
b = Singleton()
print (id(a))
print (id(b))
|
以上就是python如何实现单例模式的详细内容,更多文章请关注木庄网络博客!!
相关阅读 >>
Python实现可变变量名
Python如何遍历list
在Python中列表,数组,矩阵互相转换的方法
Python绘制五角星
最有用的Python经典书籍推荐
Python 2种方法实现叠加矩形框图层
Python第三十三天----静态方法、类方法、属性方法
为什么Python没有idle
Python模块之sys模块和序列化模块
Python解方程的技巧介绍(代码示例)
更多相关阅读请进入《Python》频道 >>
人民邮电出版社
python入门书籍,非常畅销,超高好评,python官方公认好书。
转载请注明出处:木庄网络博客 » python如何实现单例模式