本文摘自网络,作者,侵删。
今天看群里讨论mutex的实现,正好学习到这里,基本go.15的源码写了一些个人意见,错误之处欢迎留言指正哈。
// A Mutex is a mutual exclusion lock.
// The zero value for a Mutex is an unlocked mutex.
//
// A Mutex must not be copied after first use.
type Mutex struct {
state int32
sema uint32
}
// A Locker represents an object that can be locked and unlocked.
type Locker interface {
Lock()
Unlock()
}
const (
mutexLocked = 1 << iota // mutex is locked
mutexWoken
mutexStarving
mutexWaiterShift = iota
// Mutex fairness.
//
// Mutex can be in 2 modes of operations: normal and starvation.
// In normal mode waiters are queued in FIFO order, but a woken up waiter
// does not own the mutex and competes with new arriving goroutines over
// the ownership. New arriving goroutines have an advantage -- they are
// already running on CPU and there can be lots of them, so a woken up
// waiter has good chances of losing. In such case it is queued at front
// of the wait queue. If a waiter fails to acquire the mutex for more than 1ms,
// it switches mutex to the starvation mode.
//
// In starvation mode ownership of the mutex is directly handed off from
// the unlocking goroutine to the waiter at the front of the queue.
// New arriving goroutines don't try to acquire the mutex even if it appears
// to be unlocked, and don't try to spin. Instead they queue themselves at
// the tail of the wait queue.
//
// If a waiter receives ownership of the mutex and sees that either
// (1) it is the last waiter in the queue, or (2) it waited for less than 1 ms,
// it switches mutex back to normal operation mode.
//
// Normal mode has considerably better performance as a goroutine can acquire
// a mutex several times in a row even if there are blocked waiters.
// Starvation mode is important to prevent pathological cases of tail latency.
starvationThresholdNs = 1e6
)
// Lock locks m.
// If the lock is already in use, the calling goroutine
// blocks until the mutex is available.
func (m *Mutex) Lock() {
// Fast path: grab unlocked mutex.
if atomic.CompareAndSwapInt32(&m.state, 0, mutexLocked) {
if race.Enabled {
race.Acquire(unsafe.Pointer(m))
}
return
}
// Slow path (outlined so that the fast path can be inlined)
m.lockSlow()
}
func (m *Mutex) lockSlow() {
var waitStartTime int64 // 等待时间
starving := false // 是否饥饿
awoke := false // 是否唤醒
iter := 0 // 自旋次数
// state 是一个复合型的字段,
// 一个字段包含多个意义,这样可以通过尽可能少的内存来实现互斥锁。
// 这个字段的第一位(最小的一位)来表示这个锁是否被持有,
// 第二位代表是否有唤醒的 goroutine,
// 第三位代表饥饿状态,
// 剩余的位数代表的是等待此锁的 goroutine 数(阻塞等待的waiter数量)
old := m.state // 记住之前锁的状态
// 死循环阻塞住一直等获取到锁 (能够走到break退出这个循环就是拿到锁了)
for {
// Don't spin in starvation mode, ownership is handed off to waiters
// so we won't be able to acquire the mutex anyway.
// 饥饿状态下不自旋
// runtime_canSpin判断是否可以自旋,主要是多核机器 GOMAXPROCS > 1 至少有一个运行的 P 且本地队列为空
// Spin only few times and only if running on a multicore machine and
// GOMAXPROCS>1 and there is at least one other running P and local runq is empty
// iter自旋次数 < 4 (总共自旋4次)
if old&(mutexLocked|mutexStarving) == mutexLocked && runtime_canSpin(iter) {
// 非饥饿状态下的自旋逻辑
// Active spinning makes sense.
// Try to set mutexWoken flag to inform Unlock
// to not wake other blocked goroutines.
// 设置唤醒标志用来通知 Unlock 时不要唤醒其他被阻塞的 goroutines
// 还没唤醒 && 存在等待的goroutines && cas 操作成功
if !awoke && old&mutexWoken == 0 && old>>mutexWaiterShift != 0 &&
atomic.CompareAndSwapInt32(&m.state, old, old|mutexWoken) {
awoke = true
}
// 汇编实现的 PAUSE 指令(asm_amd64.s procyield)看不太懂 网上这么说
// PAUSE 指令什么都不会做,但是会消耗 CPU 时间,每次自旋都会调用 30 次 PAUSE
runtime_doSpin()
iter++
old = m.state // 再次获取锁的状态,用于后面检测锁是否被释放了
continue
}
new := old // 之前锁的状态赋值给新状态
// Don't try to acquire starving mutex, new arriving goroutines must queue.
// 不要尝试获取一个饥饿状态的锁,新来的goroutines放在等待队列里
if old&mutexStarving == 0 {
// 非饥饿 new 锁定状态即可
new |= mutexLocked
}
// 不管是锁定还是饥饿状态 waiter 数量加 1
if old&(mutexLocked|mutexStarving) != 0 {
new += 1 << mutexWaiterShift
}
// The current goroutine switches mutex to starvation mode. 当前的 g 切换 锁到饥饿模式
// But if the mutex is currently unlocked, don't do the switch. 如果当前的锁是已释放的状态就不需要切换
// Unlock expects that starving mutex has waiters, which will not
// be true in this case.
// 已经饥饿 并且 之前的锁还是未释放
if starving && old&mutexLocked != 0 {
// 设置饥饿状态
new |= mutexStarving
}
if awoke {
// The goroutine has been woken from sleep,
// so we need to reset the flag in either case.
// 不管什么情况 g 从 sleep被唤醒 都需要清除唤醒标记
if new&mutexWoken == 0 {
throw("sync: inconsistent mutex state")
}
new &^= mutexWoken // 新状态清除唤醒标记
}
// cas 操作设置锁的新状态覆盖老状态 现在老状态就是刚刚的新状态
if atomic.CompareAndSwapInt32(&m.state, old, new) {
if old&(mutexLocked|mutexStarving) == 0 {
// 锁已释放并且不是饥饿状态,代表其他 g 调用了Unlock 正常请求到了锁 直接返回
// 判断饥饿状态是为了接着走下去 照顾已经饥饿的 g 不然极端情况每次都被新来的 g 拿到锁 饥饿的就完全阻塞住了
break // locked the mutex with CAS
}
// If we were already waiting before, queue at the front of the queue.
// 如果以前就在等待了,加入到队列头
queueLifo := waitStartTime != 0
if waitStartTime == 0 {
// 没有等待时间 将当前时间复制给 等待时间
waitStartTime = runtime_nanotime()
}
// 阻塞等待
// runtime_SemacquireMutex 会在方法中循环不断调用 goparkunlock 将当前 Goroutine 陷入休眠等待信号量可以被获取就break
// goparkunlock -> gopark -> mcall(park_m)->park_m -> schedule(新的调度循环)
runtime_SemacquireMutex(&m.sema, queueLifo, 1)
// Unlock 或者正常调度 都可能唤醒
// 调度唤醒之后检查锁是否应该处于饥饿状态 等待时间超过 1 ms
starving = starving || runtime_nanotime()-waitStartTime > starvationThresholdNs
old = m.state
// 如果锁已经处于饥饿状态,直接抢到锁,返回
if old&mutexStarving != 0 {
// If this goroutine was woken and mutex is in starvation mode,
// ownership was handed off to us but mutex is in somewhat
// inconsistent state: mutexLocked is not set and we are still
// accounted as waiter. Fix that.
if old&(mutexLocked|mutexWoken) != 0 || old>>mutexWaiterShift == 0 {
throw("sync: inconsistent mutex state")
}
// 加锁并且将waiter数减1
delta := int32(mutexLocked - 1<<mutexWaiterShift)
// 最后一个waiter或者已经不饥饿了,清除饥饿标记
if !starving || old>>mutexWaiterShift == 1 {
// Exit starvation mode.
// Critical to do it here and consider wait time.
// Starvation mode is so inefficient, that two goroutines
// can go lock-step infinitely once they switch mutex
// to starvation mode.
delta -= mutexStarving
}
atomic.AddInt32(&m.state, delta)
break
}
// 唤醒之后 不是饥饿状态 进入下一次循环 尝试获取锁
awoke = true
// 自旋次数清 0
iter = 0
} else {
//cas 操作设置锁的新状态覆盖老状态 失败了 老状态不变进行下一次循环
old = m.state
}
}
if race.Enabled {
race.Acquire(unsafe.Pointer(m))
}
}
// Unlock unlocks m.
// It is a run-time error if m is not locked on entry to Unlock.
//
// A locked Mutex is not associated with a particular goroutine.
// It is allowed for one goroutine to lock a Mutex and then
// arrange for another goroutine to unlock it.
func (m *Mutex) Unlock() {
if race.Enabled {
_ = m.state
race.Release(unsafe.Pointer(m))
}
// Fast path: drop lock bit.
// 直接使用 atomic 包提供的 AddInt32,如果返回的新状态不等于 0 就会进入 unlockSlow 方法
new := atomic.AddInt32(&m.state, -mutexLocked)
if new != 0 {
// Outlined slow path to allow inlining the fast path.
// To hide unlockSlow during tracing we skip one extra frame when tracing GoUnblock.
m.unlockSlow(new)
}
}
func (m *Mutex) unlockSlow(new int32) {
// 先会对锁的状态进行校验,如果当前互斥锁已经被解锁过了就会直接抛出异常
if (new+mutexLocked)&mutexLocked == 0 {
throw("sync: unlock of unlocked mutex")
}
if new&mutexStarving == 0 {
// 非饥饿状态
old := new
for {
// If there are no waiters or a goroutine has already
// been woken or grabbed the lock, no need to wake anyone.
// In starvation mode ownership is directly handed off from unlocking
// goroutine to the next waiter. We are not part of this chain,
// since we did not observe mutexStarving when we unlocked the mutex above.
// So get off the way.
// 如果当前互斥锁不存在等待者或者最低三位表示的状态都为 0,那么当前方法就不需要唤醒其他 Goroutine 可以直接返回
if old>>mutexWaiterShift == 0 || old&(mutexLocked|mutexWoken|mutexStarving) != 0 {
return
}
// Grab the right to wake someone.
// 当有 Goroutine 正在处于等待状态时,还是会通过 runtime_Semrelease 唤醒对应的 Goroutine 并移交锁的所有权
new = (old - 1<<mutexWaiterShift) | mutexWoken
if atomic.CompareAndSwapInt32(&m.state, old, new) {
runtime_Semrelease(&m.sema, false, 1)
return
}
old = m.state
}
} else {
// 饥饿状态 直接调用 runtime_Semrelease 方法直接将当前锁交给下一个正在尝试获取锁的等待者
// 调用链 runtime_Semrelease -> sync_runtime_Semrelease -> semrelease1 ->
// readyWithTime -> goready -> ready将 g 放到 p的runnext下次调度优先执行
// 注意 handoff 为 true 的话 会调用 goyield 主动让出当前调度 这样就能尽快运行等待的 g
// Starving mode: handoff mutex ownership to the next waiter, and yield
// our time slice so that the next waiter can start to run immediately.
// Note: mutexLocked is not set, the waiter will set it after wakeup.
// But mutex is still considered locked if mutexStarving is set,
// so new coming goroutines won't acquire it.
runtime_Semrelease(&m.sema, true, 1)
}
}
本文来自:简书
感谢作者:会写点代码的萌新运维
查看原文:Golang Mutex 源码解析
相关阅读 >>
利用Go实现快看漫画网页版自动点击“下一话”,中间无需手动操作,但有一点疑惑,望大佬们解答。
【Gocn酷Go推荐】Goroutine 泄漏防治神器 Goleak
聊聊dubbo-Go-proxy的recoveryfilter
更多相关阅读请进入《Go》频道 >>
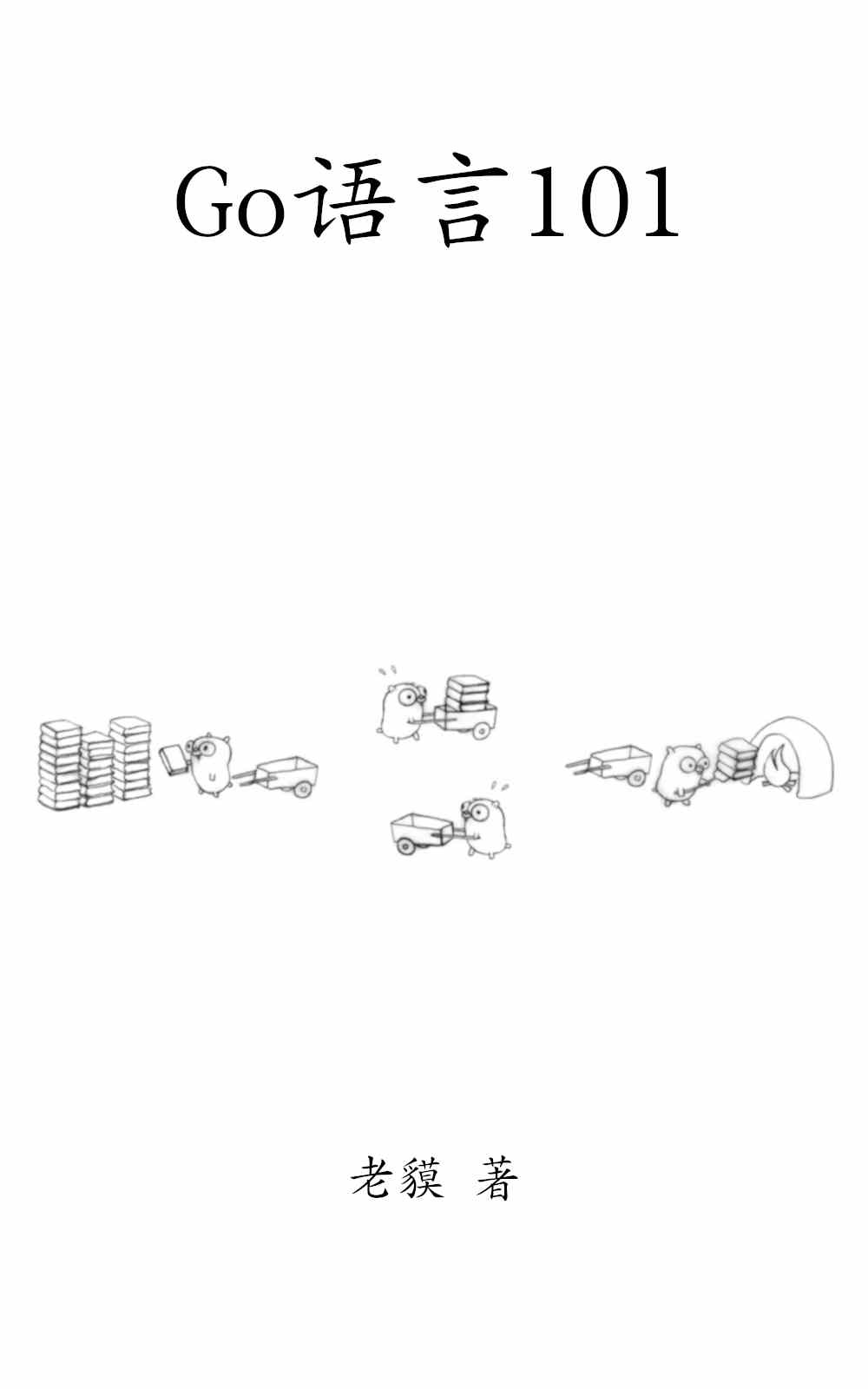
Go语言101
一个与时俱进的Go编程知识库。