本文摘自PHP中文网,作者一个新手,侵删。
经过几天的努力终于搞好了,这个类可以不用管数据库字段,不用写dao类,直接map添加,添加删除和修改,jdbc链接,分享给大家,用的话记得加上连接池,尊重原创,转载请注明
package jdbc;import java.sql.Connection;import java.sql.DriverManager;import java.sql.PreparedStatement;import java.sql.ResultSet;import java.util.ArrayList;import java.util.HashMap;import java.util.List;import java.util.Map;/** * 操作数据库工具类 * * */public class domain { /** * 连接数据 * * @return conn */ public static Connection getConnection(String url, String username, String password) { Connection conn = null; try { Class.forName("com.mysql.jdbc.Driver"); conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/" + url, username, password); } catch (Exception e) { e.printStackTrace(); } return conn; } /** * 关闭连接对象 * * @param conn * 连接对象 * @param pstmt * 预编译对象 * @param rs * 结果集 */ public static void closeAll(Connection conn, PreparedStatement pstmt, ResultSet rs) { try { if (rs != null) { rs.close(); } if (pstmt != null) { pstmt.close(); } if (conn != null) { conn.close(); } } catch (Exception e) { e.printStackTrace(); } } /** * 增删改操作 * * @param sql * SQL命令 * @param param * 参数 * @return */ public static int executUpdate(Connection conn, String sql, Object[] param) { int result = 0; PreparedStatement pstmt = null; try { System.out.println(sql); pstmt = conn.prepareStatement(sql); if (param != null) { for (int i = 0; i < param.length; i++) { pstmt.setObject(i + 1, param[i]); } } result = pstmt.executeUpdate(); } catch (Exception e) { e.printStackTrace(); } finally { closeAll(conn, pstmt, null); } return result; } /** * 查询 * * @return int * @date 2015-7-25 上午11:10:06 */ public static ResultSet executQuery(Connection conn, String sql, String[] param) { PreparedStatement pstmt = null; ResultSet result = null; try { pstmt = conn.prepareStatement(sql); if (param != null) { for (int i = 0; i < param.length; i++) { pstmt.setString(i + 1, param[i]); } } result = pstmt.executeQuery(); } catch (Exception e) { e.printStackTrace(); } return result; } /** * 简单增删改SQL语句执行,复杂sql执行executUpdate方法 * 字符串类型需要加单引号 * @param url * 数据库 * @param username * 数据库用户名 * @param password * 数据库密码 * @param map * 参数列,值 * @param tableName * 表名 * @param typeSql * SQL类型 insert,dell,update * @param oldLine * 删除时为要删除的列名,更新的时候为where前的条件列名(仅当且使用dell和update时填写,insert时不作用) * @param newLine * 更新时where候的条件列名(仅当且使用update时填写,dell,insert时不作用) * @param oldCondition * 删除时为要删除的条件,更新的时候为where前的条件(仅当且使用dell和update时填写,insert时不作用) * @param newCondition * 更新的时候为where后的条件(仅当且使用update时填写,dell,insert时不作用) */ public static void sql(String url, String username, String password, Map<String, Object> map, String tableName, String typeSql, String oldLine, String oldCondition, String newLine, String newCondition) { String sql = ""; Connection conn = getConnection(url, username, password); Object[] valueArray = null; if (typeSql.equals("insert")) { List<Object> key = new ArrayList<Object>(); List<Object> value = new ArrayList<Object>(); StringBuffer sb = new StringBuffer(); StringBuffer wen = new StringBuffer(); for (Object string : map.keySet()) { key.add(string); value.add(map.get(string)); } Object[] keyArray = key.toArray(); valueArray = value.toArray(); for (int i = 0; i < keyArray.length; i++) { sb.append(keyArray[i] + ","); wen.append("?,"); } String string = sb.toString(); string = string.substring(0, string.length() - 1); String wenStr = wen.toString(); wenStr = wenStr.substring(0, wenStr.length() - 1); sql = "INSERT INTO " + tableName + "(" + string + ") VALUES (" + wenStr + ")"; } else if (typeSql.equals("dell")) { sql = "DELETE FROM " + tableName + " WHERE " + oldLine + " = " + oldCondition; } else if (typeSql.equals("update")) { sql = "UPDATE " + tableName + " SET " + oldLine + "= " + oldCondition + " WHERE " + newLine + " = " + newCondition; } int executUpdate = executUpdate(conn, sql + ";", valueArray); System.out.println(executUpdate); }//测试 public static void main(String[] args) { Map<String, Object> map = new HashMap<String, Object>(); map.put("appid", "lisi"); for (int i = 0; i < 100; i++) { System.out.println(i); map.put("id",i); // 增加 sql("test", "root", "", map, "user", "insert", "", "", "", ""); // 删除 sql("test", "root", "", map, "user", "dell", "id", i+"","", ""); // 修改// sql("test", "root", "", map, "user", "update", "appid", "'zhang'", "id", "0"); } // map.put("password", "123"); } }
以上就是数据库jdbc封装的详细内容,更多文章请关注木庄网络博客!
相关阅读 >>
如何用mysqladministrator备份mysql数据库
怎么修改mysql root密码?修改数据库root密码的步骤
更多相关阅读请进入《JDBC》频道 >>
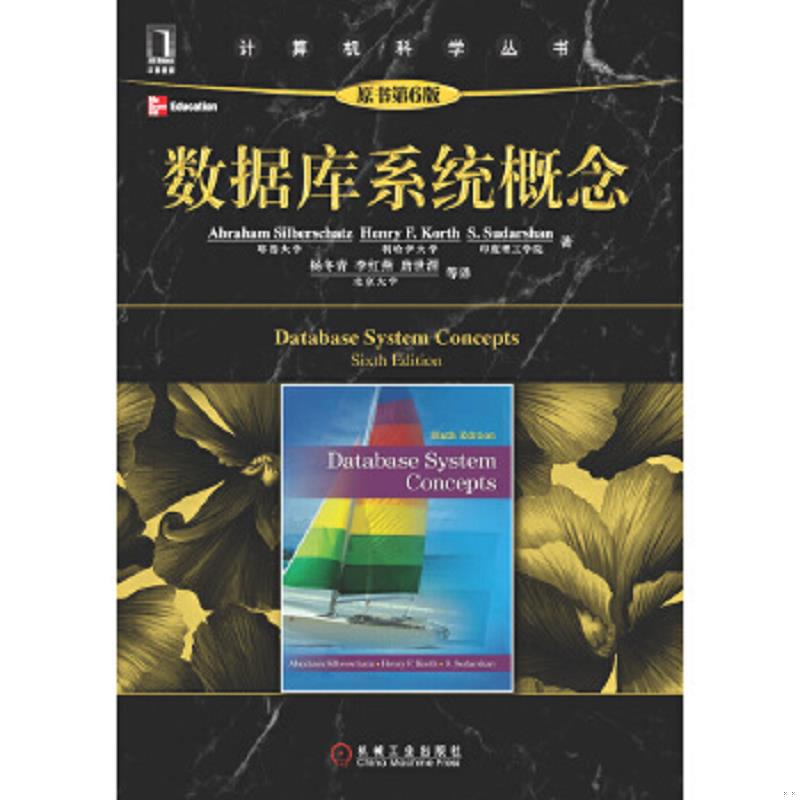
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。