本文摘自PHP中文网,作者V,侵删。

文章背景
我们的目的是在用户下单后,规定指定时间后自动将订单设置为“已过期”,不能再发起支付。
(学习视频分享:redis视频教程)
思路:
结合Redis的订阅、发布和键空间通知机制(Keyspace Notifications)进行实现。
配置redis.confg
notify-keyspace-events选项默认是不启用,改为notify-keyspace-events “Ex”。重启生效,索引位i的库,每当有过期的元素被删除时,向**keyspace@:expired**频道发送通知。
E表示键事件通知,所有通知以__keyevent@__:expired为前缀;
x表示过期事件,每当有过期被删除时发送。
与SpringBoot进行集成
1、注册JedisConnectionFactory
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
|
2、注册监听器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
3、配置订阅对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
|
paymentDataBase 库元素过期后就会跳入PaymentListener 的onMessage(Message message, byte[] pattern)方法。
相关推荐:redis数据库教程
以上就是redis实现订单自动过期功能的源码分享的详细内容,更多文章请关注木庄网络博客!
相关阅读 >>
更多相关阅读请进入《Redis》频道 >>
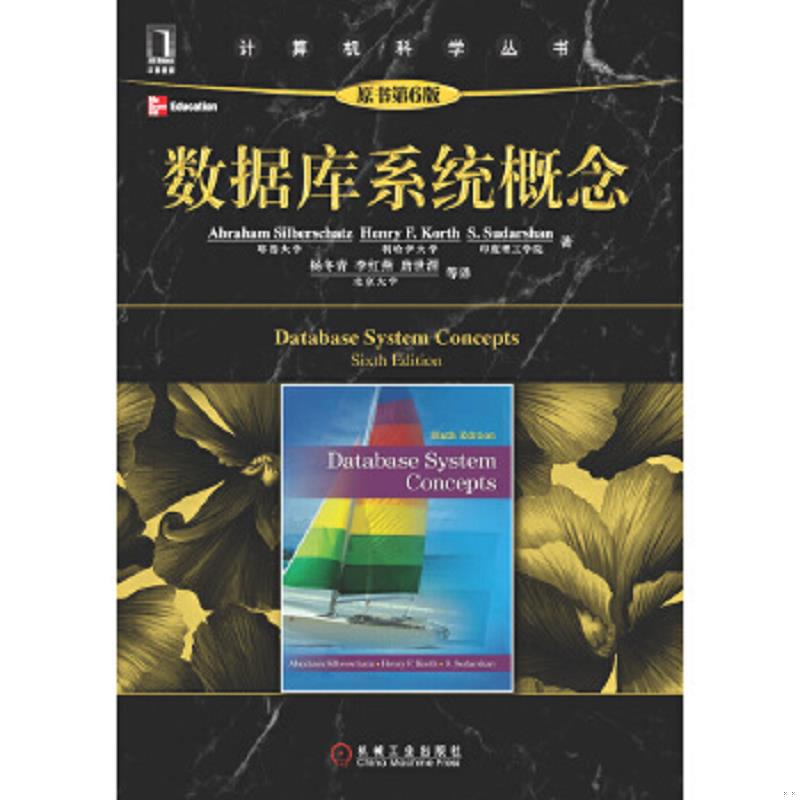
数据库系统概念 第6版
本书主要讲述了数据模型、基于对象的数据库和XML、数据存储和查询、事务管理、体系结构等方面的内容。